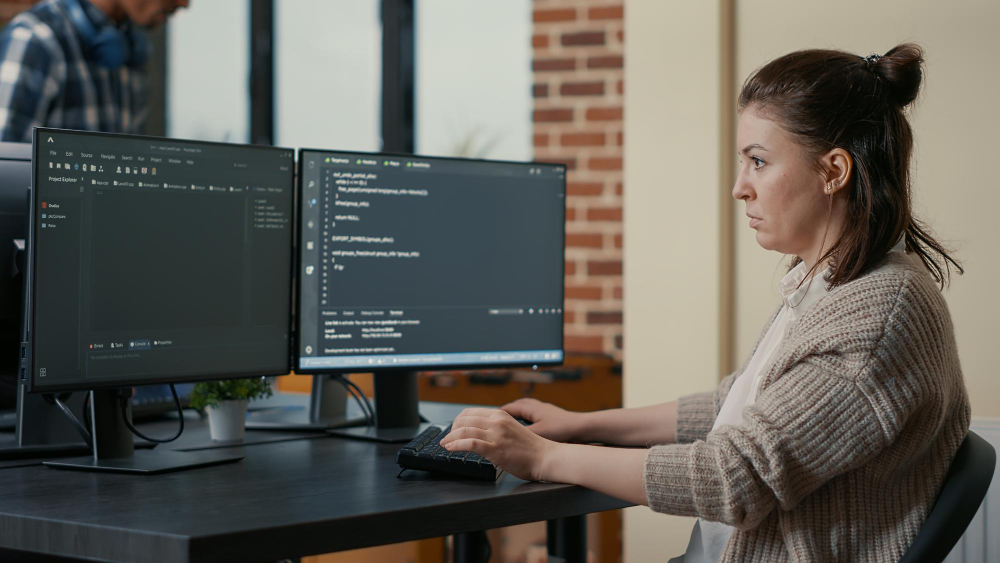
In this article, we’ll see Laravel Middleware.
Table of Contents
Laravel, the popular PHP framework, provides developers with a robust set of tools to build powerful and secure web applications. One such feature is middleware, a versatile component that sits between a request and a response, allowing you to perform various tasks and add additional layers of functionality to your application.
We’ll explore the world of Laravel middleware and discover how it can supercharge your applications by enhancing security, handling authentication, optimizing performance, and more.
What is Laravel Middleware
In Laravel, Middleware is the mechanism to apply any type of filtering you may need to HTTP requests to your application.
Laravel Middleware works between request and response of our application. It sits between Request and Response like a firewall that examines whether to route a request.Laravel comes with several inbuilt middlewares like EncryptCookies ,RedirectIfAuthenticated,TrimStrings,TrustProxies etc .
All middleware in Laravel is created in the Middleware folder, located inside the app/HTTP
folder.
A few things I could think of that you could achieve through middleware are:
- logging of requests
- redirecting users
- altering/sanitizing the incoming parameters
- manipulating the response generated by the Laravel application
- and many more
Creating Middleware
We can easily create new Laravel middleware using artisan.
php artisan make:middleware AdMiddleware
After we’ve created a new middleware component, we then need to look at modifying the code to suit our needs.
Updating our Middleware File:
After you’ve run the make: middleware command you should see your new middleware file in app/http/middleware. Open this up and we’ll create a middleware that will get the request’s IP address and then determine what country that request came from.
<?php
namespace App\Http\Middleware;
use Closure;
class AdMiddleware
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
// Test to see if the requesters have an ip address.
if($request->ip() == null){
throw new \Exception("IP ADDRESS NOT SET");
}
$country=file_get_contents('http://api.hostip.info/get_html.php?ip=' . $request->ip());
echo $country;
if(strpos($country, "UNITED STATES")){
throw new \Exception("NOT FOR YOUR EYES, NSA");
} else {
return redirect("index");
}
return $next($request);
}
}
This code basically takes in a request and as an example checks to see its location before deciding whether to display a US-only ad or an advertisement suited for the rest of the world. This could be quite beneficial for those of you who want to build up a site that features amazon affiliate links from multiple countries.
Note that you shouldn’t be passing anything back in the middleware section, you should instead be passing redirects to views instead of printing out things like I’ve done for brevity.
Registering your Middleware
When registering your Laravel middleware you have 2 choices. The first choice is that you add the middleware to be run on every request handled by your app. You can do that by opening up App\Http\Kernel.php and adding it to your $middleware array like so:
protected $middleware = [
\Illuminate\Foundation\Http\Middleware\CheckForMaintenanceMode::class,
\App\Http\Middleware\EncryptCookies::class,
\Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class,
\Illuminate\Session\Middleware\StartSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\App\Http\Middleware\VerifyCsrfToken::class,
// our new class.
\App\http\Middleware\AdMiddleware::class,
];
Second choice is to have the middleware run on registered routes only, you can register it like so:
<?php
/**
* The application's route middleware.
*
* @var array
*/
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class,
'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class,
'ad' => \App\Http\Middleware\AdMiddleware::class,
];
And then add the middleware to the specific routes like so:
Route::get('/ip', ['middleware' => 'ad', function() {
return "IP";
}]);
Laravel middleware empowers developers to add layers of functionality, enhance security, optimize performance, and handle various aspects of the request-response lifecycle. By understanding the power and flexibility of middleware, you can unlock a world of possibilities in your Laravel applications.
Whether it’s securing routes, manipulating requests and responses, optimizing performance, or implementing custom logic, middleware is a powerful tool that will elevate your Laravel development experience to new heights.
I hope this article helps!