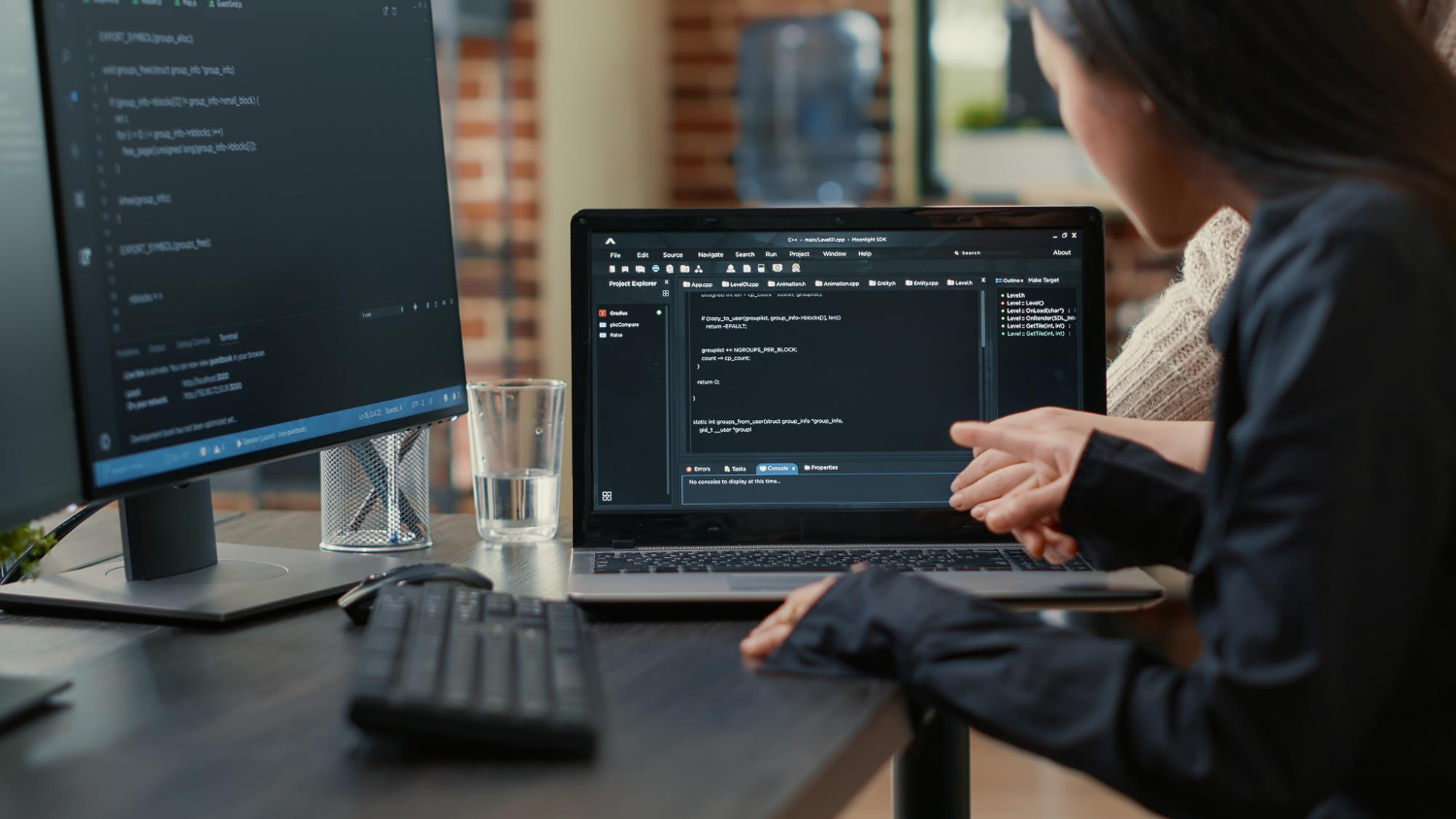
In the world of Laravel, controllers play a pivotal role in organizing and managing the application’s logic. They act as intermediaries between routes and views, handling user requests, processing data, and returning appropriate responses.
Table of Contents
Mastering Laravel controllers is essential for building robust and maintainable applications. In this comprehensive guide, we’ll delve into the intricacies of Laravel controllers, exploring best practices, advanced techniques, and practical examples to empower developers to wield this powerful tool effectively.
Understanding Laravel Controllers
Laravel follows the MVC (Model-View-Controller) architectural pattern, where controllers serve as the C (Controller) component.
Laravel Controllers are PHP classes responsible for processing incoming HTTP requests and organizing the application’s response. They encapsulate the application’s logic, facilitating the separation of concerns and promoting code reusability.
In Laravel, controllers are like the managers of the application. They receive requests from the routes and decide what should happen next. The controller can get data from a database or an API, pass it to the view, or manipulate it in another way.
Laravel Controllers can group related request-handling logic into a single class. For example, a UserController
class might handle all incoming requests related to users, including showing, creating, updating, and deleting users.
By default, Laravel controllers are stored in the app/Http/Controllers
directory.
Creating Laravel Controllers:
Creating controllers in Laravel is a straightforward process. Developers can generate controllers using the artisan command-line tool or manually create them within the app/Http/Controllers directory.
Artisan simplifies the creation process by generating boilerplate code, saving time and effort. Once created, controllers can be associated with specific routes, defining the actions to be performed when a particular route is accessed.
To create a controller in Laravel, you can use the artisan command-line tool. Navigate to your project directory and run the following command:
php artisan make:controller UserController
This command will generate a UserController class within the app/Http/Controllers
directory.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class UserController extends Controller { // }
Routing and Controller Actions
Routing plays a crucial role in connecting HTTP requests to controller actions. Laravel’s routing system allows developers to define routes that map to specific controller methods.
Controller actions are PHP functions within the controller class, each responsible for handling a particular HTTP request. By defining routes and associating them with controller actions, developers can create a clear and organized structure for handling incoming requests.
Routes in Laravel are defined in the routes/web.php
file. You can associate routes with controller actions using the Route::
facade.
use App\Http\Controllers\UserController; Route::get('/users', [UserController::class, 'index']); Route::post('/users', [UserController::class, 'store']); Route::get('/users/{id}', [UserController::class, 'show']); Route::put('/users/{id}', [UserController::class, 'update']); Route::delete('/users/{id}', [UserController::class, 'destroy']);
In the UserController
, you would define methods corresponding to these actions:
class UserController extends Controller { public function index() { // Logic to fetch and return all users } public function store(Request $request) { // Logic to store a new user } public function show($id) { // Logic to fetch and return a specific user } public function update(Request $request, $id) { // Logic to update a specific user } public function destroy($id) { // Logic to delete a specific user } }
Middleware and Controller Middleware
Middleware provides a convenient mechanism for filtering HTTP requests entering the application. In Laravel, middleware can be applied at various levels, including globally, route-specific, or controller-specific.
Controller middleware allows developers to apply middleware to a group of controller actions, providing a centralized location for specifying request filters and enhancing security, authentication, and validation.
Middleware can be applied to controller actions by specifying it in the controller’s constructor or using the middleware()
method within the controller.
You can also apply middleware only to specific actions within a controller by using the only()
or except()
methods.
namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Http\Controllers\Controller; class UserController extends Controller { public function __construct() { $this->middleware('auth')->except(['index', 'show']); } }
Here, the ‘auth’ middleware will be applied to all controller actions except index
and show
.
Resource Controllers
Resource controllers provide a convenient way to implement CRUD operations for a resource in Laravel. They adhere to RESTful design principles, offering standardized endpoints for creating, reading, updating, and deleting resource instances.
To create a resource controller in Laravel, you can use the make:controller
artisan command with the --resource
flag:
php artisan make:controller UserController --resource
This command generates a UserController with methods for handling various CRUD operations.
Laravel provides a concise way to map resourceful routes to controller actions using the Route::resource()
method:
use App\Http\Controllers\UserController; Route::resource('users', UserController::class);
This single line of code generates the following routes:
GET /users
: Index (display a list of resources)GET /users/create
: Create (display a form for creating a new resource)POST /users
: Store (store a newly created resource)GET /users/{user}
: Show (display a specific resource)GET /users/{user}/edit
: Edit (display a form for editing a specific resource)PUT/PATCH /users/{user}
: Update (update a specific resource)DELETE /users/{user}
: Destroy (delete a specific resource)
In the UserController, each method corresponds to a resource action. For example:
namespace App\Http\Controllers; use App\Models\User; use Illuminate\Http\Request; class UserController extends Controller { public function index() { $users = User::all(); return view('users.index', ['users' => $users]); } public function create() { return view('users.create'); } public function store(Request $request) { // Validate and store the new user } public function show(User $user) { return view('users.show', ['user' => $user]); } public function edit(User $user) { return view('users.edit', ['user' => $user]); } public function update(Request $request, User $user) { // Validate and update the user } public function destroy(User $user) { $user->delete(); return redirect()->route('users.index'); } }
While Laravel provides default resourceful routes, you can customize them by specifying only the required routes using the only()
or except()
methods:
Route::resource('users', UserController::class)->only(['index', 'show']);
This will only generate routes for the index and show actions.
References:
Credits:
Conclusion:
The Laravel controllers are the backbone of web application development. They handle requests and generate responses in a structured manner. By learning how to use Laravel controllers correctly and following best practices, you will be able to create applications that are fast, easy to maintain, and scalable.
Laravel has a wide range of features and an easy-to-use interface. This makes it easy for developers to develop complex web applications. With the help of the Laravel controllers, you can take your development skills to the next level.
The techniques and principles mentioned in this guide will help you get the most out of your Laravel controllers.