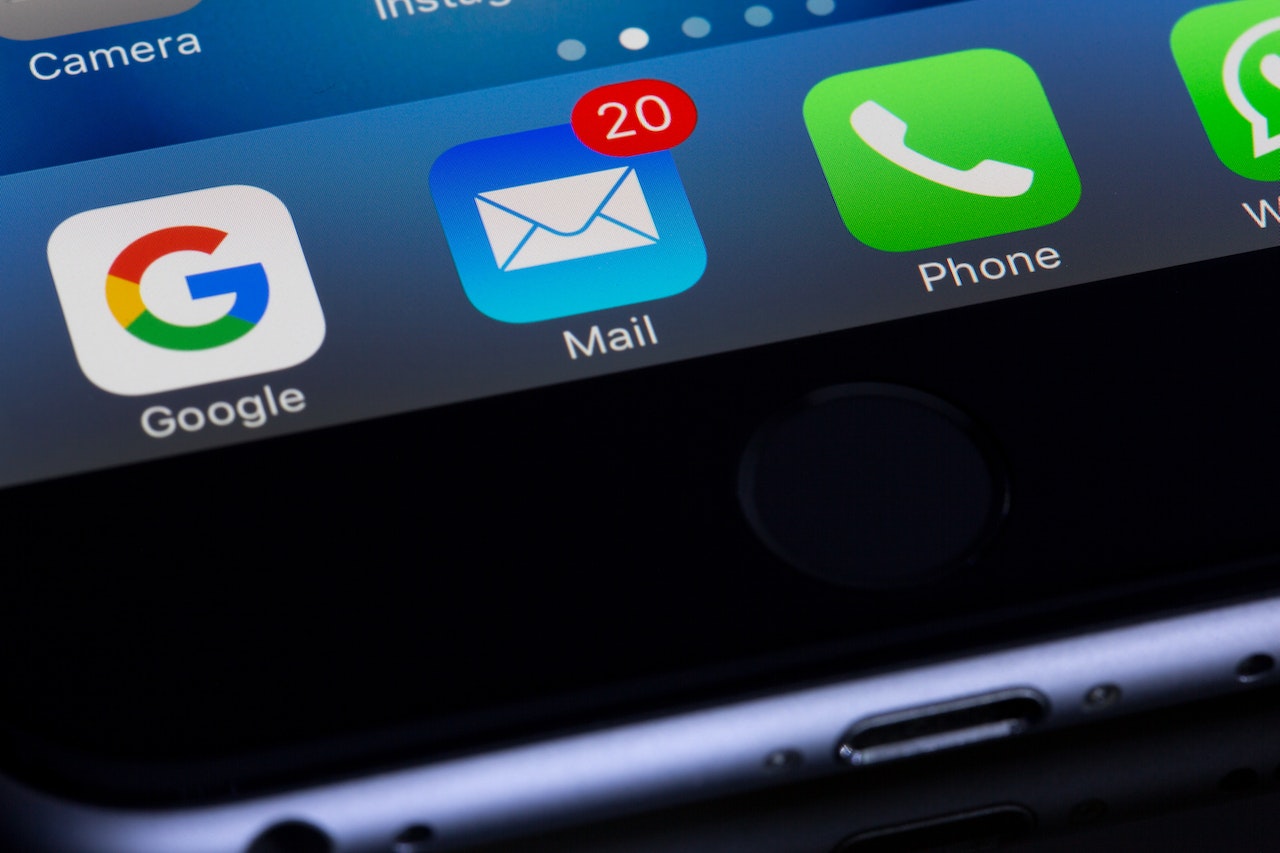
In this article, we will learn how to send Laravel Email Notifications. Laravel provides an easy way of sending an email using the Notification class.
Table of Contents
Building meaningful connections with your users is crucial in today’s digital landscape. Laravel, a friendly PHP framework, offers an intuitive and efficient email notification system that simplifies the process of sending automated emails.
We’ll dive into the world of Laravel email notifications, exploring their benefits and demonstrating how you can leverage them to create engaging user experiences on your web applications.
Laravel Email Notifications
Laravel Email notifications are a powerful tool for keeping users informed, engaged, and connected to your web application. Laravel’s email notification system streamlines the process, making it a breeze to send personalized and timely messages. Whether it’s welcoming new users, sending order updates, or providing password reset instructions, Laravel empowers you to deliver seamless communication that resonates with your audience.
Benefits of Laravel Email Notifications:
- Personal Touch: Laravel email notifications enable you to craft personalized messages based on user actions or events. Tailoring your emails adds a human touch, fostering a stronger connection and enhancing the user experience.
- Time Efficiency: Automating email notifications with Laravel saves you precious time. Instead of manually composing and sending emails, you can rely on Laravel’s built-in features to handle the delivery process, freeing you up to focus on other essential tasks.
- Consistent Branding: With Laravel, you can ensure consistent branding across all email communications. Customizable email templates allow you to maintain your brand’s visual identity, reinforcing recognition and building trust among your users.
Implementing Laravel Email Notifications:
The advantage of using Laravel email notifications is that this is already associated with the specified user we just put a parameter of User object value then the class will handle the sending.
Follow the below steps:
1). First you need to create a Laravel app. you can create an app with the following artisan command:
composer create-project --prefer-dist laravel/laravel laravel-email-notification
2) Once you created an app, you need to create a table to save notifications in the database. you need to run the following two artisan commands to create and migrate the table:
php artisan notifications:table
php artisan migrate
3). Now the table is created. you need to create a notification class using the following command:
php artisan make:notification EmailNotification
Once, you run the above command in the terminal then a notification file called “EmailNotification” is created at the “App/Notifications” directory. for example, see below:
<?php namespace App\Notifications; use Illuminate\Bus\Queueable; use Illuminate\Notifications\Notification; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Notifications\Messages\MailMessage; class EmailNotification extends Notification { use Queueable; private $details; /** * Create a new notification instance. * * @return void */ public function __construct($details) { $this->details = $details; } /** * Get the notification's delivery channels. * * @param mixed $notifiable * @return array */ public function via($notifiable) { return ['mail','database']; } /** * Get the mail representation of the notification. * * @param mixed $notifiable * @return \Illuminate\Notifications\Messages\MailMessage */ public function toMail($notifiable) { return (new MailMessage) ->greeting($this->details['greeting']) ->line($this->details['body']) ->action($this->details['actionText'], $this->details['actionURL']) ->line($this->details['thanks']); } /** * Get the array representation of the notification. * * @param mixed $notifiable * @return array */ public function toDatabase($notifiable) { return [ 'order_id' => $this->details['order_id'] ]; } }
4). Now, you need to create a route to send notifications. Just open the “routes/web.php” file and add the following routes.
Route::get('send', 'NotificationController@send');
5). Now, you need to create a controller called “NotificationController” which is defined in routes. you can create a controller with following command:
php artisan make:controller NotificationController
6). Now, open the “NotificationController.php” controlled file inside app/Http/Controllers/
directory and add the following code
<?php namespace App\Http\Controllers; use Notification; use App\Models\User; use Illuminate\Http\Request; use App\Notifications\EmailNotification; class NotificationController extends Controller { public function send() { $user = User::first(); $project = [ 'greeting' => 'Hi '.$user->name.',', 'body' => 'This is my message.', 'thanks' => 'Thank you', 'actionText' => 'View', 'actionURL' => url('/'), 'order_id' => 1]; Notification::send($user, new EmailNotification($project)); dd('Notification sent!'); } }
7). Now, the application is ready. Run the following two commands to open the app and send the notification:
php artisan serve
http://127.0.0.1:8000/send
Laravel email notifications empower you to create engaging user experiences by delivering timely and personalized communication. With its intuitive features, you can automate email delivery, infuse a personal touch, and maintain consistent branding. Leverage the power of Laravel to establish strong connections with your users, keeping them informed, engaged, and excited about your web applications. Let Laravel handle the complexities of Laravel email notifications while you focus on building meaningful relationships and delighting your users.
I hope this article helps!