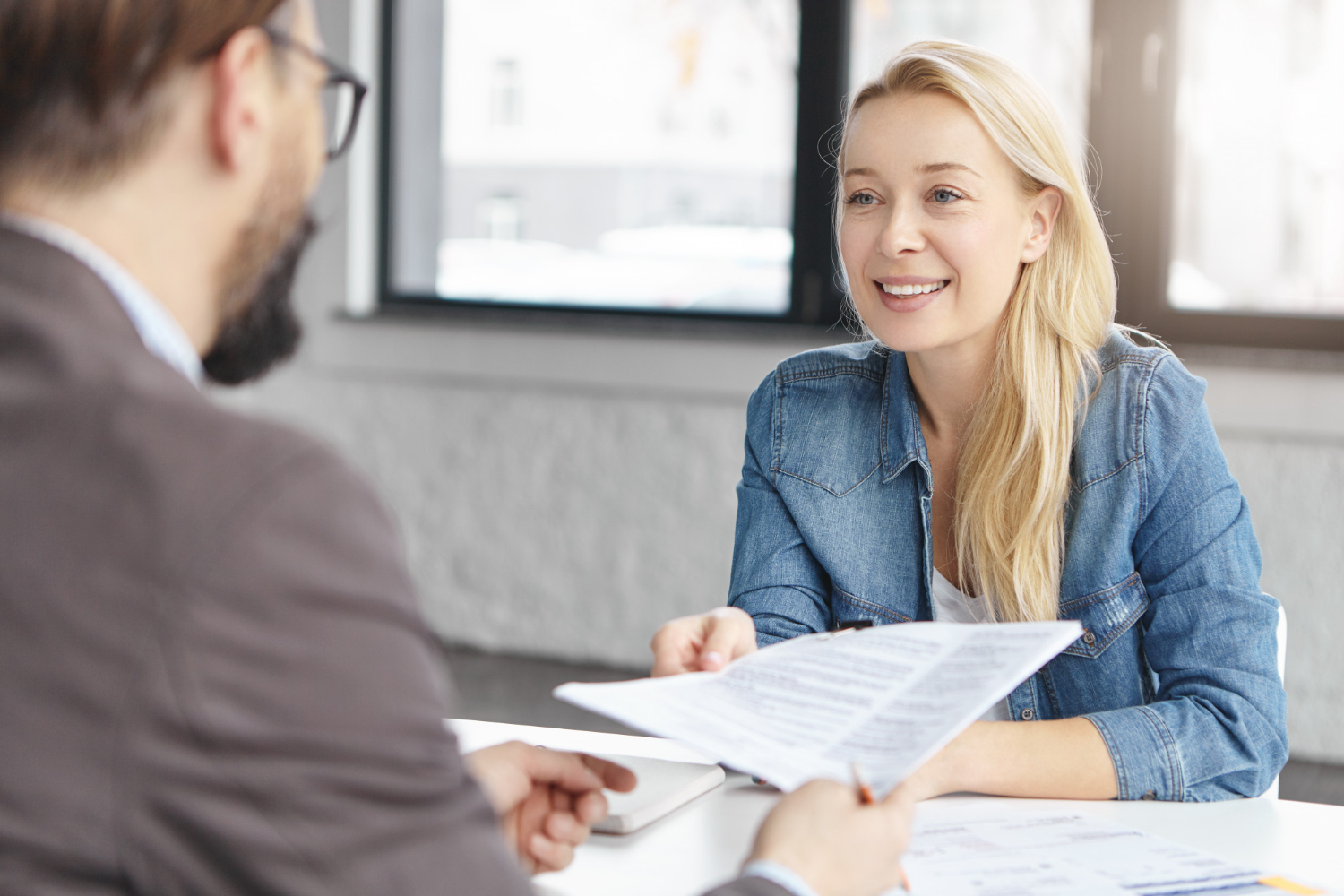
JavaScript Interview Questions are a critical part of technical assessments, as JavaScript continues to dominate the development world. It’s essential for web development, both on the client and server side. To help you prepare for your JavaScript interviews, here is a list of the top 24 most commonly asked javascript interview questions with detailed answers and references for further reading
List of Most Asked JavaScript Interview Questions
JavaScript is a lightweight, interpreted, or JIT-compiled language with the following features:
- Dynamic typing
- First-class functions
- Prototypal inheritance
- Event-driven and non-blocking model (via asynchronous programming)
- Cross-platform compatibility
In JavaScript interview questions about variable declarations, it’s important to distinguish between let, const, and var:
- var: Function-scoped, can be redeclared, and allows hoisting.
- let: Block-scoped, cannot be redeclared in the same scope.
- const: Block-scoped, must be initialized during declaration, and its value cannot be reassigned.
When tackling JavaScript interview questions about equality operators, you need to understand that:
==
: Performs type coercion before comparing values.===
: Strict equality, compares both value and type.
Example:
console.log(5 == '5'); // true console.log(5 === '5'); // false
JavaScript interview questions about closures often test your understanding of functions and scope. A closure is a function that remembers its outer variables even after the outer function has executed.
Example:
function outer() { let count = 0; return function inner() { count++; return count; }; } const counter = outer(); console.log(counter()); // 1 console.log(counter()); // 2
Event delegation is a popular topic in JavaScript interview questions. It’s a technique for adding an event listener to a parent element instead of individual child elements. It utilizes event bubbling to capture events at the parent level.
Example:
document.getElementById('parent').addEventListener('click', (event) => { if (event.target.tagName === 'BUTTON') { console.log('Button clicked:', event.target.textContent); } });
JavaScript interview questions about programming models often focus on the distinction between synchronous and asynchronous approaches:
- Synchronous: Code is executed sequentially, blocking further execution until the current task completes.
- Asynchronous: Code can execute without waiting for a task to complete, using callbacks, promises, or
async/await
.
Promises frequently come up in JavaScript interview questions about asynchronous operations. A Promise is an object representing the eventual completion or failure of an asynchronous operation. It has three states: pending, fulfilled, and rejected.
Example:
let promise = new Promise((resolve, reject) => { setTimeout(() => resolve('Success!'), 1000); }); promise.then(console.log).catch(console.error);
JavaScript interview questions on function methods often include call(), apply(), and bind():
call()
: Invokes a function with a specificthis
value and arguments individually.apply()
: Invokes a function with a specificthis
value and arguments as an array.bind()
: Returns a new function with a boundthis
value.
Example:
const obj = { name: 'John' }; function greet(greeting) { return `${greeting}, ${this.name}`; } console.log(greet.call(obj, 'Hello')); // Hello, John console.log(greet.apply(obj, ['Hi'])); // Hi, John const boundGreet = greet.bind(obj); console.log(boundGreet('Hey')); // Hey, John
JavaScript interview questions about data types often explore null and undefined:
null
: Explicitly represents the absence of value.undefined
: Indicates a variable has been declared but not initialized.
Example:
let x; console.log(x); // undefined console.log(null); // null
In JavaScript interview questions about code quality, you might encounter use strict. It enforces stricter parsing and error handling in JavaScript code, preventing common mistakes like using undeclared variables.
Example:
'use strict'; x = 10; // Throws ReferenceError
Hoisting is a common topic in JavaScript interview questions about execution contexts. It refers to JavaScript’s behavior of moving variable and function declarations to the top of their scope before code execution.
Example:
console.log(a); // undefined a = 10; var a;
Arrow functions are a shorthand syntax for writing functions. They do not have their own this
, arguments
, or super
and cannot be used as constructors.
Example:
const add = (a, b) => a + b; console.log(add(2, 3)); // 5
Prototypes are objects from which other objects inherit properties and methods. Every JavaScript object has a prototype, accessible via __proto__
.
Example:
function Person(name) { this.name = name; } Person.prototype.greet = function() { return `Hello, ${this.name}`; };
- Shallow Copy: Copies the top-level properties only.
- Deep Copy: Copies all levels of an object.
Example:
const obj1 = { a: 1, b: { c: 2 } }; const shallowCopy = { ...obj1 }; const deepCopy = JSON.parse(JSON.stringify(obj1));
JavaScript modules allow developers to break code into reusable pieces. They use import
and export
statements to share code between files.
Example:
// module.js export const add = (a, b) => a + b; // main.js import { add } from './module.js'; console.log(add(2, 3)); // 5
The this
keyword refers to the object that is executing the current function. It varies depending on how the function is called.
Example:
const obj = { name: 'Alice', greet() { console.log(`Hello, ${this.name}`); } }; obj.greet(); // Hello, Alice
Event bubbling is when an event propagates from a child element up to its parent elements, while event capturing is the reverse, where it propagates from the parent down to the child element.
Example:
document.querySelector('#child').addEventListener('click', () => { console.log('Child clicked'); }, true); // Capturing phase document.querySelector('#parent').addEventListener('click', () => { console.log('Parent clicked'); }, false); // Bubbling phase
async/await
is a syntactic sugar built on Promises that makes asynchronous code look and behave like synchronous code.
Example:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error:', error); } } fetchData();
JavaScript has eight data types: seven primitives (string
, number
, bigint
, boolean
, undefined
, symbol
, null
) and one non-primitive (object
).
Example:
let str = 'Hello'; let num = 42; let bigInt = 123n; let bool = true; let undef; let sym = Symbol('id'); let obj = {};
map()
: Returns a new array with the results of applying a callback function to each element.forEach()
: Executes a callback function for each element without returning a new array.
Example:
const arr = [1, 2, 3]; const mapped = arr.map(x => x * 2); // [2, 4, 6] arr.forEach(x => console.log(x));
A higher-order function is a function that takes another function as an argument or returns a function as a result.
Example:
function higherOrder(fn) { return function(arg) { return fn(arg) * 2; }; } const double = higherOrder(x => x + 3); console.log(double(5)); // 16
A generator function is a special type of function that can pause execution and resume later, allowing for iterative processing.
Example:
function* generator() { yield 1; yield 2; yield 3; } const gen = generator(); console.log(gen.next().value); // 1 console.log(gen.next().value); // 2
The typeof
operator returns the data type of a variable or expression.
Example:
console.log(typeof 'Hello'); // "string" console.log(typeof 42); // "number"
Destructuring allows unpacking values from arrays or properties from objects into distinct variables.
Example:
const arr = [1, 2, 3]; const [a, b] = arr; console.log(a, b); // 1, 2 const obj = { x: 10, y: 20 }; const { x, y } = obj; console.log(x, y); // 10, 20
Credits
Conclusion
JavaScript is an incredibly versatile language, and mastering its core concepts is essential for any web developer. The questions listed above, ranging from basic syntax to advanced concepts like closures and asynchronous programming, are designed to prepare you for success in interviews. Ensure you practice writing code and explore the provided references to deepen your understanding. Best of luck in your JavaScript interview preparation!