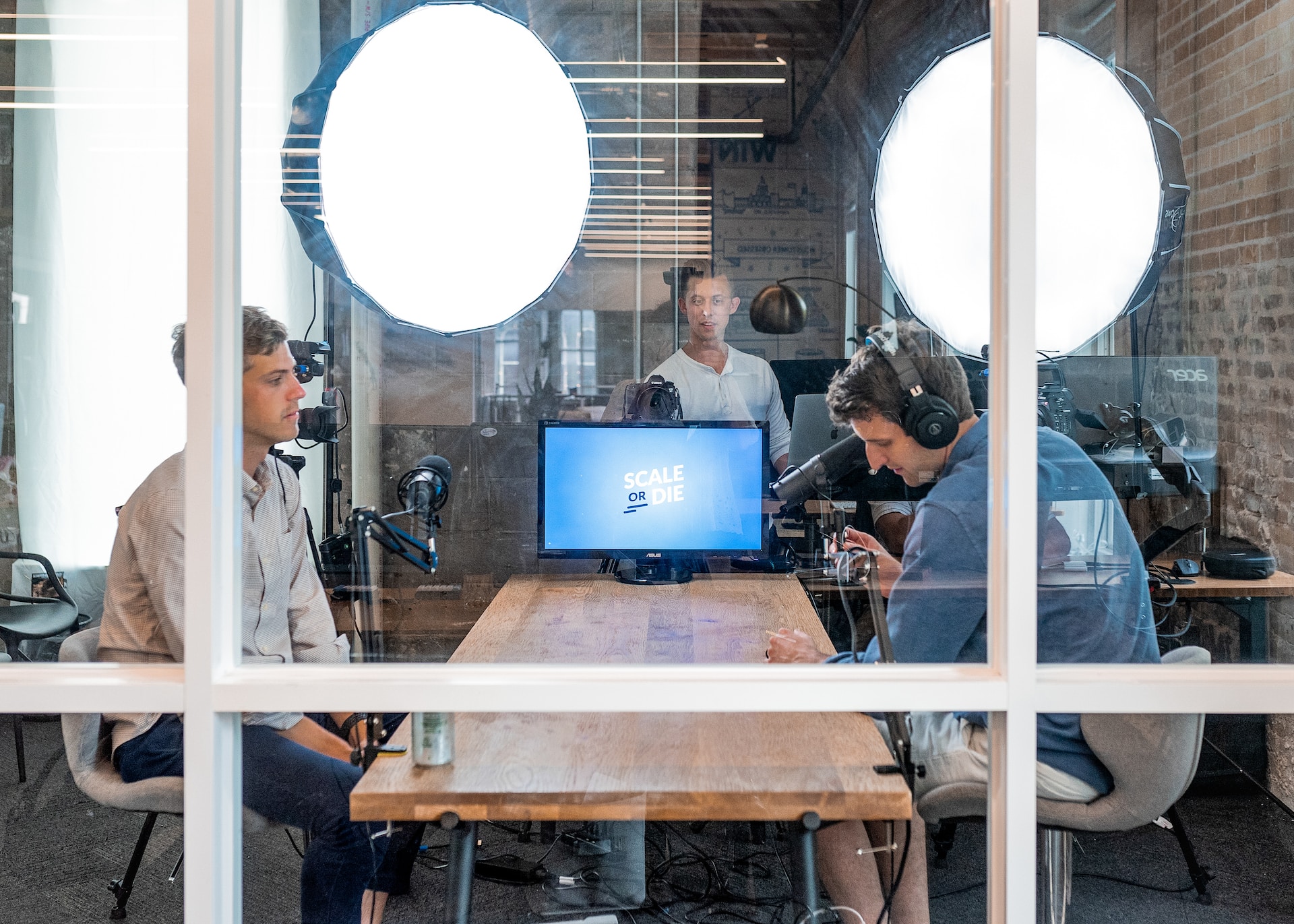
In this article, we’ll see list of Node JS Interview Questions.
Node.js is a popular open-source runtime environment that uses JavaScript to execute code outside of a browser. It is used to develop a wide variety of applications, including web applications, real-time applications, and data-intensive applications.
Its event-driven, non-blocking I/O model has revolutionized the world of web development. As a result, Node.js developers are in high demand, and the interview process can be quite challenging.
To help you prepare effectively, we have compiled a list of ten unique Node js interview questions that will test your knowledge and showcase your expertise in this powerful JavaScript runtime.
Node JS Interview Questions
see below node js interview questions with answers:
Node.js is a very powerful JavaScript-based platform or framework which is built on Google Chrome’s JavaScript V8 Engine.
Node.js was developed in 2009 by Ryan Dahl.
4) What are the features of Node.js?
Below are the features of Node.js –
- Very Fast
- Event-driven and Asynchronous
- Single-Threaded but highly Scalable
5) Explain REPL in Node.js?
REPL stands for Read Eval Print Loop. Node.js comes with bundled REPL environment which performs the following desired tasks –
- Eval
- Loop
- Read
6) Explain variables in Node.js?
Variables are used to store values and print later like any conventional script. If the “var” keyword is used then the value is stored in a variable. You can print the value in the variable using – console.log().
Eg: $ node > a = 30 30 > var b = 50 undefined > a + b 80 > console.log("Hi") Hi undefined
7) What is the latest version of Node.js available?
The latest version of Node.js is – v0.10.36.
8) List out some REPL commands in Node.js?
Below is the list of REPL commands –
- Ctrl + c – For terminating the current command.
- Ctrl + c twice – For terminating REPL.
- Ctrl + d – For terminating REPL.
- Tab Keys – list of all the current commands.
- .break – exit from multiline expression.
- .save with filename – save REPL session to a file.
9) Mention the command to stop REPL in Node.js?
Command – ctrl + c twice is used to stop REPL.
10) Explain NPM in Node.js?
NPM stands for Node Package Manager (npm) and there are two functionalities which NPM takes care of mainly and they are –
- Online repositories for node.js modules or packages, which can be searched on search.nodejs.org
- Dependency Management, Version Management and command line utility for installing Node.js packages.
11) Mention command to verify the NPM version in Node.js?
The below command can be used to verify the NPM version –
$ npm --version
12) How you can update NPM to the new version in Node.js?
Below commands can be used for updating NPM to a new version –
$ sudo npm install npm -g /usr/bin/npm -> /usr/lib/node_modules/npm/bin/npm-cli.js npm@2.7.1 /usr/lib/node_modules/npm
13) Explain callback in Node.js?
The callback is called once the asynchronous operation has been completed. Node.js heavily uses callbacks and all APIs of Node.js are written to support callbacks.
14) How Node.js can be made more scalable?
Node.js works well for I/O bound and not CPU bound work. For instance, if there is a function to read a file, file reading will be started during that instruction and then it moves onto the next instruction, and once the I/O is done or completed it will call the callback function. So there will not be any blocking.
15) Explain global installation of dependencies?
Globally installed dependencies or packages are stored in <user-directory>/npm directory and these dependencies can be used in Command Line Interface function of any node.js.
16) Explain local installation of dependencies?
This will be present in the root directory of any Node module/application and will be used to define the properties of a package.
“Streams” are objects which will let you read the data from the source and write data to the destination as a continuous process.
20) What do you mean by chaining in Node.JS?
It’s a mechanism in which the output of one stream will be connected to another stream and thus creating a chain of multiple stream operations.
21) Explain the Child process module?
The child process module has the following three major ways to create child processes –
- spawn – child_process.spawn launches a new process with a given command.
- exec – child_process.exec method runs a command in a shell/console and buffers the output.
- fork – The child_process.fork method is a special case of the spawn() to create child processes.
22) Why to use exec method for the Child process module?
“exec” method runs a command in a shell and buffers the output. Below is the command –
child_process.exec(command[, options], callback)
23) List out the parameters passed for Child process module?
Below are the list of parameters passed for Child Process Module –
child_process.exec(command[, options], callback)
- command – This is the command to run with space-separated arguments.
- options – This is an object array which comprises one or more following options –
- cwd
- uid
- gid
- killSignal
- maxBuffer
- encoding
- env
- shell
- timeout
callback – This is the function which is gets 2 arguments – stdout, stderr and error.
24) What is the use of method – “spawn()”?
This method is used to launch a new process with the given commands. Below is the method signature –
child_process.spawn(command[, args][, options])
25) What is the use of method – “fork()”?
This method is a special case for method- “spawn()” for creating node processes. The method signature –
child_process.fork(modulePath[, args][, options])
26) Explain Piping Stream?
This is a mechanism of connecting one stream to other and this is basically used for getting the data from one stream and pass the output of this to other stream.
27) What would be the limit for Piping Stream?
There will not be any limit for piping stream.
28) Explain FS module ?
Here FS stands for “File System” and fs module is used for File I/O. FS module can be imported in the following way –
var test = require("fs")
29) Explain “Console” in Node.JS?
“Console” is a global object and will be used for printing to stderr and stdout and this will be used in synchronous manner in case of destination is either file or terminal or else it is used in asynchronous manner when it is a pipe.
30) Explain – “console.log([data][, …])” statement in Node.JS?
This statement is used for printing to “stdout” with newline and this function takes multiple arguments as “printf()”.
31) What you mean by “process”?
- Fatal Error
- Non-function Internal Exception Handler
- Internal JavaScript Parse Error
- Uncaught Fatal Exception
- Unused
- Internal JavaScript Evaluation Failure
- Internal Exception Handler Run-Time Failure
- Platform
- Stdin
- Stdout
- Stderr
- execPath
- mainModule
- execArgv
- config
- arch
- title
- version
- argv
- env
- exitCode
34) Define OS module?
OS module is used for some basic operating system related utility functions. Below is the syntax for importing OS module –
var MyopSystem = require("os")
35) What is the property of OS module?
os.EOL – Constant for defining appropriate end of line marker for OS.
36) Explain “Path” module in Node.JS?
“Path” module will be used for transforming and handling file paths. Below is the syntax of path module –
var mypath = require("path")
37) Explain “Net” module in Node.JS?
“Net” module is being used for creating both clients and servers. It will provide asynchronous network wrapper. Below is the syntax of Net module –
var mynet = require("net")
38) List out the differences between AngularJS and NodeJS?
AngularJS is a web application development framework. It’s a JavaScript and it is different from other web app frameworks written in JavaScript like jQuery. NodeJS is a runtime environment used for building server-side applications while AngularJS is a JavaScript framework mainly useful in building/developing client-side part of applications which run inside a web browser.
39) NodeJS is client side server side language?
NodeJS is a runtime system, which is used for creating server-side applications.
40) What are the advantages of NodeJS?
Below are the list of advantages of NodeJS –
- Javascript – It’s a javascript which can be used on frontend and backend.
- Community Driven – NodeJS has great open source community which has developed many excellent modules for NodeJS to add additional capabilities to NodeJS applications.
41) In which scenarios NodeJS works well?
NodeJS is not appropriate to use in scenarios where single-threaded calculations are going to be the holdup.
42) What you mean by JSON?
JavaScript Object Notation (JSON) is a practical, compound, widely popular data exchange format. This will enable JavaScript developers to quickly construct APIs.
43) Explain “Stub”?
Stub is a small program, which substitutes for a longer program, possibly to be loaded later and that is located remotely. Stubs are functions/programs that simulate the behaviors of components/modules.
44) List out all Node.JS versions available?
Below are the list of all NodsJS versions supported in operating systems –
OperatingSystem | Node.js version |
---|---|
Windows | node-v0.12.0-x64.msi |
Linux | node-v0.12.0-linux-x86.tar.gz |
Mac | node-v0.12.0-darwin-x86.tar.gz |
SunOS | node-v0.12.0-sunos-x86.tar.gz |
45) Explain “Buffer class” in Node.JS?
It is a global class which can be accessed in an application without importing buffer modules.
46) How we can convert Buffer to JSON?
The syntax to convert Buffer to JSON is as shown beow
buffer.toJSON()
47) How to concatenate buffers in NodeJS?
var MyConctBuffer = Buffer.concat([myBuffer1, myBuffer2]);
Mybuffer1.compare(Mybuffer2);
buffer.copy(targetBuffer[, targetStart][, sourceStart][, sourceEnd])
- readUIntBE – It’s a generalized version of all numeric read methods, which supports up to 48 bits accuracy. Setting noAssert to “true” to skip the validation.
- writeIntBE – This will write the value to the buffer at the specified byteLength and offset and it supports upto 48 bits of accuracy.
51) Why to use “__filename” in Node.JS?
“__filename” is used to represent the filename of the code which is being executed. It used to resolve the absolute path of file. Below is the sample code for the same –
Console.log(__filename);
52) Why to use “SetTimeout” in Node.JS?
This is the global function and it is used to run the callback after some milliseconds.
Syntax of this method –
setTimeout(callbackmethod, millisecs)
53) Why to use “ClearTimeout” in Node.JS?
This is the global function and it is used to stop a timer which was created during “settimeout()”.
54) Explain Web Server?
It is a software app which will handle the HTTP requests by client (eg: browser) and will return web pages to client as a response. Most of web server supports – server side scripts using scripting languages. Example of web server is Apache, which is mostly used webserver.
55) List out the layers involved in Web App Architechure?
Below are the layers used in Web Apps –
- Client – Which makes HTTP request to the server. Eg: Browsers.
- Server – This layer is used to intercept the requests from client.
- Business – It will have application server utilized by web servers for processing.
- Data – This layer will have databases mainly or any source of data.
56) Explain “Event Emitter” in Node.JS?
It is a part of Events module. When instance of EventEmitter faces any error, it will emit an ‘error’ event. “Event Emitters” provides multiple properties like – “emit” and “on”.
- “on” property is used for binding the function with event.
- “emit” property is used for firing an event.
57) Explain “NewListener” in Node.JS?
This event is being emitted whenever any listener is added. So when event is triggered the listener may not have been removed from listener array for the event.
58) Why to use Net.socket in Node.JS?
This object is an abstraction of a local socket or TCP. net.Socket instances implement a duplex Stream interface. These can be created by the user and used as a client (with connect() function) or they can be created by Node and can be passed to the user through the ‘connection’ event of a server.
59) Which events are emitted by Net.socket?
Below are the list of events emitted by Net.socket –
- Connect
- Lookup
- End
- Data
- Close
- Drain
- Timeout
- Error
60) Explain “DNS module” in Node.JS?
This module is used for DNS lookup and to use underlying OS name resolution. This used to provide asynchronous network wrapper. DNS module can be imported like –
var mydns = require("dns")
61) Explain binding in domain module in Node.JS?
Below are the bindings in domain modules –
- External Binding
- Internal Binding
62) Explain RESTful Web Service?
Web services which uses REST architecture will be known as RESTful Web Services. These web services uses HTTP protocol and HTTP methods.
63) How to truncate the file in Node.JS?
Below command can be used for truncating the file –
fs.ftruncate(fd, len, callback)
These unique Node js interview questions cover various aspects of Node js development and will enable you to showcase your knowledge and expertise.
By mastering these concepts, you’ll be better prepared to tackle challenging node js interview questions and demonstrate your ability to build scalable and efficient server-side applications with Node.js. Good luck!