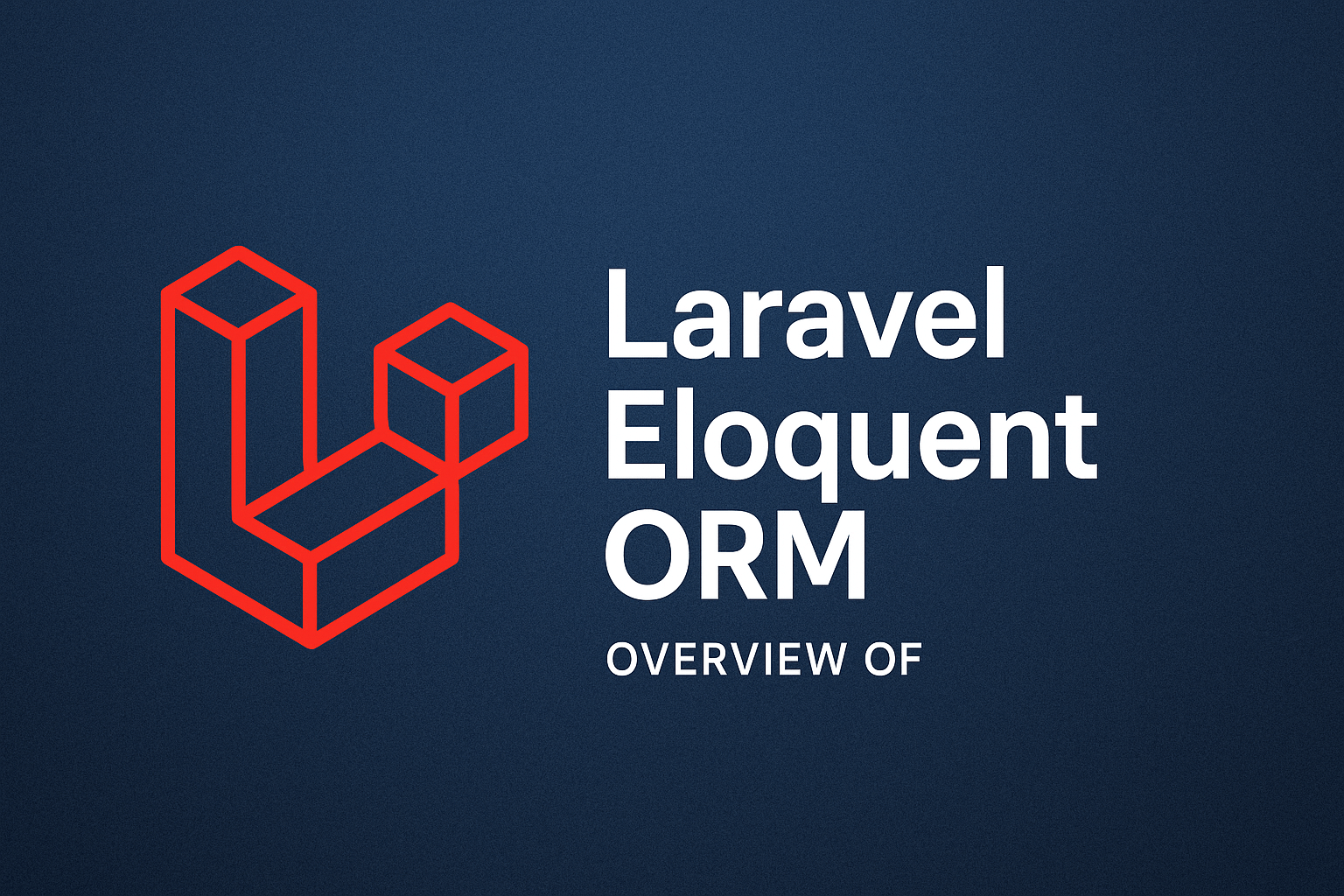
Laravel’s Eloquent ORM (Object-Relational Mapper) is one of the framework’s standout features, designed to make database interactions effortless and intuitive. It provides an elegant, simple Active Record implementation that allows developers to work with databases using PHP objects rather than writing complex SQL queries.
Table of Contents
This article explores the core concepts of Eloquent ORM, its features, and how it simplifies database management in Laravel applications.
Laravel, one of the most popular PHP frameworks, has revolutionized the way developers interact with databases through its powerful Object-Relational Mapping (ORM) tool called Eloquent ORM. Eloquent ORM simplifies database operations by allowing developers to work with databases using an intuitive, object-oriented syntax
What is Eloquent ORM?
Eloquent ORM is Laravel’s built-in ORM that bridges the gap between application logic and database tables. It represents each database table as a model class, and each record in the table is an instance of that class.
Eloquent ORM is Laravel’s implementation of the Active Record pattern, which enables developers to interact with databases using PHP objects instead of writing raw SQL queries. Each database table is represented by a corresponding “model” class, and each row in the table is represented as an instance of that model.
This abstraction makes database interaction cleaner and more maintainable by eliminating the need to write raw SQL queries for most operations.
Eloquent is a technique for querying data using the Laravel Model. In this technique, you don’t need to write a full (long) query; you just need to use some Eloquent syntax, and you can get data from complex queries.
If want to search id 1 in users table than you can use this.
$user = App\User::find(1);
Instead of.
$user = DB::table('users')->where('id', '1')->first();
Key Features of Eloquent
- Active Record Implementation: Eloquent ORM follows the Active Record pattern, which means each model corresponds to a database table. This pattern simplifies database interactions by encapsulating the logic for querying and manipulating data within the model itself.
- Intuitive Relationships: Eloquent ORM simplifies defining relationships such as one-to-one, one-to-many, and many-to-many. With these, you can easily fetch related data without manually writing JOIN queries. For example, fetching all comments for a blog post becomes straightforward.
- Query Builder Integration: Eloquent ORM works seamlessly with Laravel’s query builder, allowing developers to write flexible and readable queries. This integration ensures that even advanced queries can be built easily while maintaining clean code.
- Eager Loading: Eager loading helps optimize database queries by preloading related models in a single query. For example, instead of running separate queries for a post and its comments, eager loading combines them to improve performance.
- Mutators and Accessors: These features allow developers to manipulate attribute values when retrieving or setting them. Mutators modify data before it is saved to the database, while accessors format it after retrieval, providing cleaner and more consistent outputs.
- Event Hooks: Eloquent provides lifecycle hooks for models, such as
creating
,saving
,updating
, anddeleting
. These hooks allow developers to execute logic automatically at specific points in a model’s lifecycle.
Benefits of Using Eloquent ORM
- Improved Readability: Eloquent’s expressive syntax makes database interactions more readable and maintainable compared to raw SQL queries.
- Reduced Boilerplate Code: By abstracting common database operations, Eloquent reduces the amount of boilerplate code developers need to write.
- Enhanced Security: Eloquent automatically escapes user inputs, reducing the risk of SQL injection attacks.
- Database Agnostic: Eloquent supports multiple database systems, including MySQL, PostgreSQL, SQLite, and SQL Server, making it easy to switch between databases if needed.
- Rapid Development: With features like relationships, scopes, and eager loading, Eloquent accelerates the development process, allowing developers to focus on building features rather than writing repetitive database queries.
Eloquent ORM: Use Cases
- User Authentication: Eloquent is widely used in Laravel authentication systems, managing user registration, login, and sessions.
- Content Management Systems (CMS): Blogging platforms and CMS applications use Eloquent to handle categories, posts, comments, and users.
- E-Commerce Applications: Eloquent helps manage products, orders, customers, payments, and inventories in online stores.
- API Development: RESTful and GraphQL APIs built with Laravel use Eloquent ORM for data retrieval and manipulation.
- Data Analysis and Reporting: Eloquent enables efficient querying and filtering for analytics dashboards and reports.
Setting Up Eloquent ORM
Prerequisites
- Laravel installed (minimum version 8 recommended).
- A configured database connection in the
.env
file.
Example .env
configuration:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=secret
Creating a Model
Run the Artisan command to generate a new Eloquent model:
php artisan make:model Post
This command creates a Post
model in the app/Models
directory. By default, the model assumes there is a database table named posts
.
Defining a Table
If the table name does not follow Laravel’s naming convention, specify it explicitly in the model:
class Post extends Model { protected $table = 'blog_posts'; }
Basic CRUD Operations
Eloquent simplifies CRUD (Create, Read, Update, Delete) operations with its intuitive syntax.
Create
You can create a new record using the create
method or by instantiating the model:
// Using create() Post::create([ 'title' => 'First Post', 'content' => 'This is the content of the first post.', ]); // Using instantiation $post = new Post; $post->title = 'Second Post'; $post->content = 'This is the content of the second post.'; $post->save();
Note: Ensure the fillable
property is defined in the model to allow mass assignment.
protected $fillable = [‘title’, ‘content’];
Read
Retrieve records using methods like all
, find
, or where
:
// Get all posts $posts = Post::all(); // Find a post by ID $post = Post::find(1); // Query with conditions $posts = Post::where('title', 'like', '%Laravel%')->get();
Update
Updating records is as straightforward as retrieving and modifying them:
$post = Post::find(1);
$post->title = 'Updated Title';
$post->save();
Or, use the update
method: Post::where('id', 1)->update(['title' => 'Another Updated Title']);
Delete
Delete records using the delete
method
$post = Post::find(1); $post->delete(); // Or directly Post::destroy(2);
Defining Relationships
Eloquent makes defining relationships between models simple. Here are some common types:
One-to-One
Define a one-to-one relationship using the hasOne
and belongsTo
methods:
// User Model public function profile() { return $this->hasOne(Profile::class); } // Profile Model public function user() { return $this->belongsTo(User::class); }
This type of relationship is useful when a single entity has exactly one associated entity, such as a user having one profile.
One-to-one relationships link a single record in one table to a single record in another, ideal for entities like user profiles.
One-to-Many
// Post Model public function comments() { return $this->hasMany(Comment::class); } // Comment Model public function post() { return $this->belongsTo(Post::class); }
One-to-many relationships are ideal for scenarios where one record can have multiple associated records, like a blog post with many comments.
One-to-many relationships provide a straightforward way to manage parent-child data structures, commonly used in hierarchical datasets.
Many-to-Many
// User Model public function roles() { return $this->belongsToMany(Role::class); } // Role Model public function users() { return $this->belongsToMany(User::class); }
Many-to-many relationships allow multiple records in one table to be associated with multiple records in another table, such as users belonging to multiple roles.
Summary: Many-to-many relationships facilitate complex associations between tables, enabling flexible and dynamic data linkage.
Eager Loading
Optimize queries by preloading relationships:
$posts = Post::with('comments')->get();
Eager loading reduces the number of queries by fetching related models in a single query.
Eager loading boosts performance by minimizing database queries, crucial for optimizing relational data retrieval.
Advanced Features
Accessors and Mutators
Accessors format attributes when retrieved:
public function getTitleAttribute($value) { return strtoupper($value); }
Mutators modify attributes before saving:
public function setTitleAttribute($value) { $this->attributes['title'] = strtolower($value); }
Accessors and mutators provide a powerful way to manage data consistency and presentation within Eloquent models.
Model Events
Eloquent allows you to hook into model events:
protected static function boot() { parent::boot(); static::creating(function ($model) { // Logic before creating a model }); }
Model events offer hooks for embedding business logic during key model operations, enhancing automation and control.
Soft Deletes
Enable soft deletes by adding the SoftDeletes
trait:
use Illuminate\Database\Eloquent\SoftDeletes; class Post extends Model { use SoftDeletes; }
Run the migration to add a deleted_at
column:
Schema::table('posts', function (Blueprint $table) { $table->softDeletes(); });
Soft deletes safeguard data by marking it as deleted without actual removal, providing flexibility for recovery and audits.
Eloquent ORM Architecture
Eloquent follows the Active Record Pattern, meaning each model represents a database table and each instance represents a row in that table.
Key Components of Eloquent Architecture
- Model: Represents a table in the database
- Query Builder: Provides a fluent interface to construct database queries
- Relationships: Defines connections between different models
- Eager Loading: Optimizes queries by reducing database calls
- Mutators & Accessors: Transform data when setting or retrieving attributes
- Events & Observers: Handle model event-driven actions
Eloquent ORM Workflow
- Define a model that extends
Illuminate\Database\Eloquent\Model
. - Configure properties like
$table
,$fillable
, and$guarded
. - Use relationships to manage associations between models.
- Perform database operations using Eloquent’s API.
Credits:
- Photo by Mohammad Rahmani on Unsplash
Best Practices
- Use Relationships: Leverage Eloquent relationships for clean and maintainable code.
- Limit Queries: Avoid the N+1 query problem by using eager loading.
- Validate Data: Always validate input data before passing it to the model.
- Follow Conventions: Stick to Laravel’s naming conventions for smooth development.
- Optimize Queries: Use indexes and efficient queries for better performance.
Conclusion
Eloquent ORM is a game-changer for Laravel developers, offering a seamless and intuitive way to interact with databases. Its rich feature set, combined with Laravel’s elegant syntax, makes it an indispensable tool for building modern web applications. By following best practices and leveraging Eloquent’s capabilities, developers can write cleaner, more efficient, and secure code.
Whether you’re a beginner or an experienced developer, mastering Eloquent ORM will undoubtedly enhance your productivity and elevate the quality of your Laravel projects.