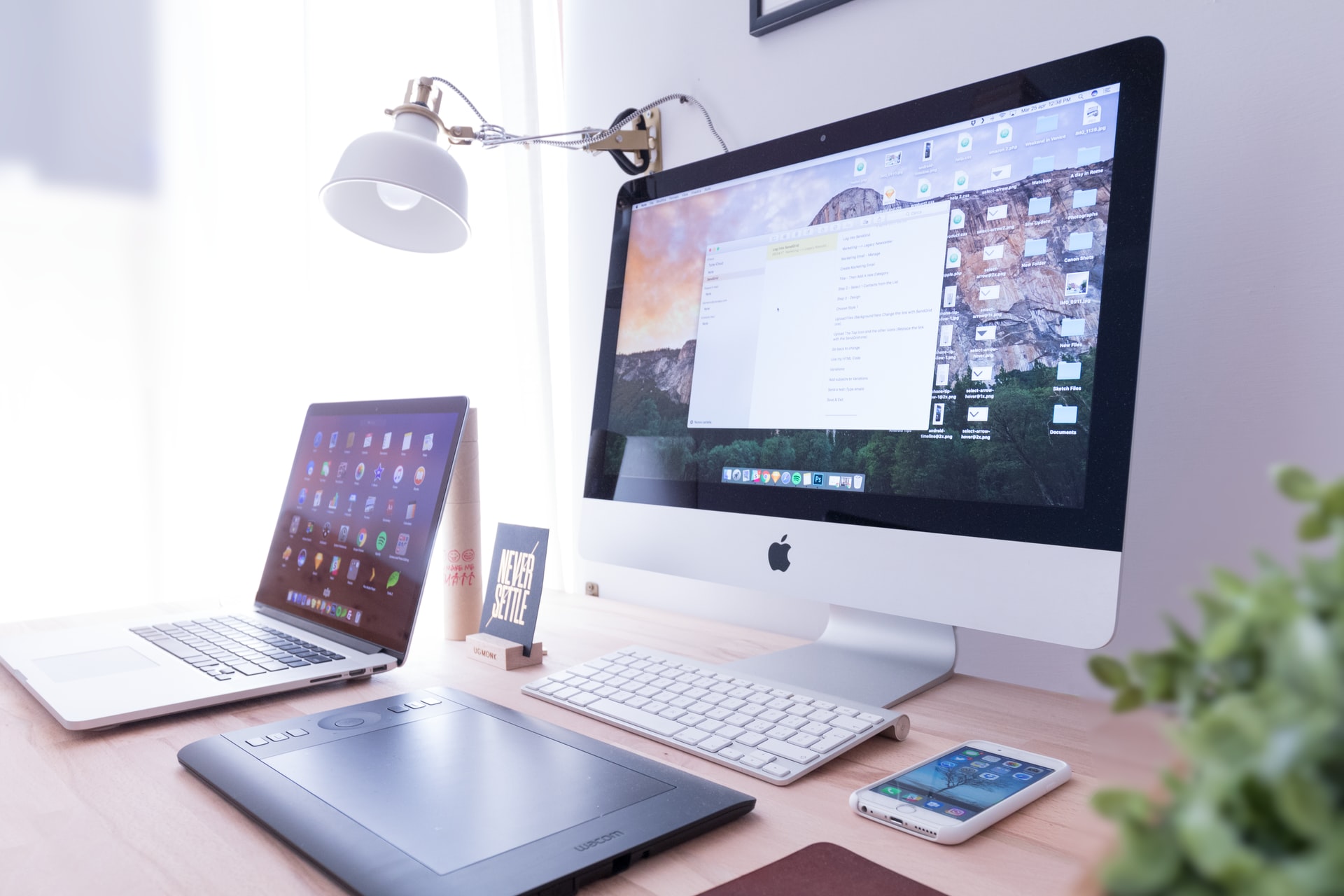
In this article, we’ll Create Web Service With PHP JSON and MySql.
Table of Contents
In today’s interconnected world, web services play a crucial role in enabling seamless communication and data exchange between applications.
Building a robust and efficient web service requires careful consideration of various technologies.
We will explore how to create a powerful web service with PHP, JSON, and MySQL, three popular technologies that work together seamlessly to deliver dynamic and data-driven web applications.
What are Web services
Web services ( application services ) are one of the most essential parts of today’s development where we centralize data and allow the user to access that data from different sources like the web, software, app, etc. Web services provide Interoperability between two different languages. Web services are easy to understand or to make we can easily create a web service for our website. There are number of methods through which you can create your web service
- SOAP { Simple Object Access Protocol }.
- REST { Representational State Transfer }
We are going to create a Web Service With PHP using the REST method but I also give you a small overview of the SOAP method first.
What is SOAP?.
SOAP is a Simple Object Access Protocol based on XML so it is easy to read. It is a simple XML-based protocol to exchange data between two different languages.
What is REST?.
REST { Representational State Transfer } is a simple stateless architecture that generally runs over HTTP. REST web service system produces status code responses in JSON or XML format.
Creating web services using REST is very easy and takes less time to make as compared to others.
REST support all the most commonly used HTTP methods (GET, POST, PUT, and DELETE). We use all these methods according to need.
Create Web Service With PHP JSON and MySql
Application using REST
Now I am going to create a small application using REST. In this application, we can create a SignUp, Get user Info and Update user status. Before creating this application it is recommended that you have a basic understanding of PHP, MYSQL, JSON. I later explain to you how we can use this in Android to access data from web service with PHP.
Step 1. I hope that you have already installed WAMP, Laragon, or XAMPP on your computer.
Step 2. Now we need to install a chrome extension for testing or web service so I use Advance REST Client.
REST Client is very useful to test or web services. You simply follow the link i mention above and install the extension in your Chrome browser.
Step 3. Now we are going to create our database http://localhost/phpmyadmin .
— — Database: `tuts_rest` — create database IF NOT EXISTS `tuts_rest` — ——————————————————– — — Table structure for table `users` — CREATE TABLE IF NOT EXISTS `users` ( `ID` int(11) NOT NULL AUTO_INCREMENT, `name` text NOT NULL, `email` varchar(100) NOT NULL, `password` varchar(100) NOT NULL, `status` text NOT NULL, PRIMARY KEY (`ID`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
Copy-paste this sql query to your PHPMyAdmin-> sql.
Step 4. Now we need to create a data handler file in php and url where we can handle request information.
Code: confi.php
<?php $conn = mysql_connect("localhost", "root", ""); mysql_select_db('tuts_rest', $conn);
first, we need to connect to our database. Now we can create a file to save requested data to data base
signup.php
Requested URL: http://localhost/aneh/rest/signup.php
<?php // Include confi.php include_once(‘confi.php’); if($_SERVER[‘REQUEST_METHOD’] == “POST”){ // Get data $name = isset($_POST[‘name’]) ? mysql_real_escape_string($_POST[‘name’]) : “”; $email = isset($_POST[’email’]) ? mysql_real_escape_string($_POST[’email’]) : “”; $password = isset($_POST[‘pwd’]) ? mysql_real_escape_string($_POST[‘pwd’]) : “”; $status = isset($_POST[‘status’]) ? mysql_real_escape_string($_POST[‘status’]) : “”; // Insert data into data base $sql = “INSERT INTO `tuts_rest`.`users` (`ID`, `name`, `email`, `password`, `status`) VALUES (NULL, ‘$name’, ‘$email’, ‘$password’, ‘$status’);”; $qur = mysql_query($sql); if($qur){ $json = array(“status” => 1, “msg” => “Done User added!”); }else{ $json = array(“status” => 0, “msg” => “Error adding user!”); } }else{ $json = array(“status” => 0, “msg” => “Request method not accepted”); } @mysql_close($conn); /* Output header */ header(‘Content-type: application/json’); echo json_encode($json);
In the signup page I did not add any validation you can add your validation here if you like the main and important thing on this page is
header(‘Content-type: application/json’);
we tell php that this page is return as json, and we also use json_encode() to return our data in json format.
Now after this we need Advance Rest Client to send data to our page. Go to your chrome app store where you add your extensions
click on Advance Rest client. Now add your request
url: http://localhost/aneh/rest/signup.php, Select the Request method, Click on Add new vaule define your value and data in it and click on send.
after doing all this you need send data to server and this output like this
That all if you get done message!.
Now we get user info using GET method. I create a new php file to get user info once you have command on this you easily work on single page.
Info.php
Requester URL: url: http://localhost/aneh/rest/info.php?uid=Request_ID
<?php // Include confi.php include_once(‘confi.php’); $uid = isset($_GET[‘uid’]) ? mysql_real_escape_string($_GET[‘uid’]) : “”; if(!empty($uid)){ $qur = mysql_query(“select name, email, status from `users` where ID=’$uid'”); $result =array(); while($r = mysql_fetch_array($qur)){ extract($r); $result[] = array(“name” => $name, “email” => $email, ‘status’ => $status); } $json = array(“status” => 1, “info” => $result); }else{ $json = array(“status” => 0, “msg” => “User ID not define”); } @mysql_close($conn); /* Output header */ header(‘Content-type: application/json’); echo json_encode($json);
In this, we pass user id in URL. Add this URL in Advance REST Client and click on send and get output like this:
{ status: 1 info: [1] 0: { name: "aneh tahkur" email: "anehkuamr@gmail.com" status: "Cool!!" }- - }
Now we can update user info using the PUT method. To read about the Put method follow this link.
status.php
Request URL: http://localhost/aneh/rest/status.php
Update user status
<?php // Include confi.php include_once(‘confi.php’); if($_SERVER[‘REQUEST_METHOD’] == “PUT”){ $uid = isset($_SERVER[‘HTTP_UID’]) ? mysql_real_escape_string($_SERVER[‘HTTP_UID’]) : “”; $status = isset($_SERVER[‘HTTP_STATUS’]) ? mysql_real_escape_string($_SERVER[‘HTTP_STATUS’]) : “”; // Add your validations if(!empty($uid)){ $qur = mysql_query(“UPDATE `tuts_rest`.`users` SET `status` = ‘$status’ WHERE `users`.`ID` =’$uid’;”); if($qur){ $json = array(“status” => 1, “msg” => “Status updated!!.”); }else{ $json = array(“status” => 0, “msg” => “Error updating status”); } }else{ $json = array(“status” => 0, “msg” => “User ID not define”); } }else{ $json = array(“status” => 0, “msg” => “User ID not define”); } @mysql_close($conn); /* Output header */ header(‘Content-type: application/json’); echo json_encode($json);
In the above I am using the PUT method in PUT we access data like this: $_SERVER[‘HTTP_DATAVARIABLE’].
Sending PUT Request from Advanced Rest Client.
In this, we add a URL where we want to make a request and then select Request method PUT and then add the header as shown in the above image.
The output of above is
{ status: 1 msg: “Status updated!!.” }
Creating a powerful web service with PHP, JSON, and MySQL empowers you to build dynamic and data-driven applications with ease.
By leveraging the strengths of each technology, you can develop robust APIs that allow seamless communication and data exchange.
Remember to follow best practices for security, input validation, and error handling to ensure the reliability and security of your web service with PHP.
With the knowledge gained from this blog post, you’re ready to embark on your journey to build sophisticated and efficient Web Service With PHP. Happy coding!
This is a straightforward and easy example web service with PHP, JSON, and MySQL-based example.
I simply must tell you that you have an excellent and unique site that I really enjoyed reading.