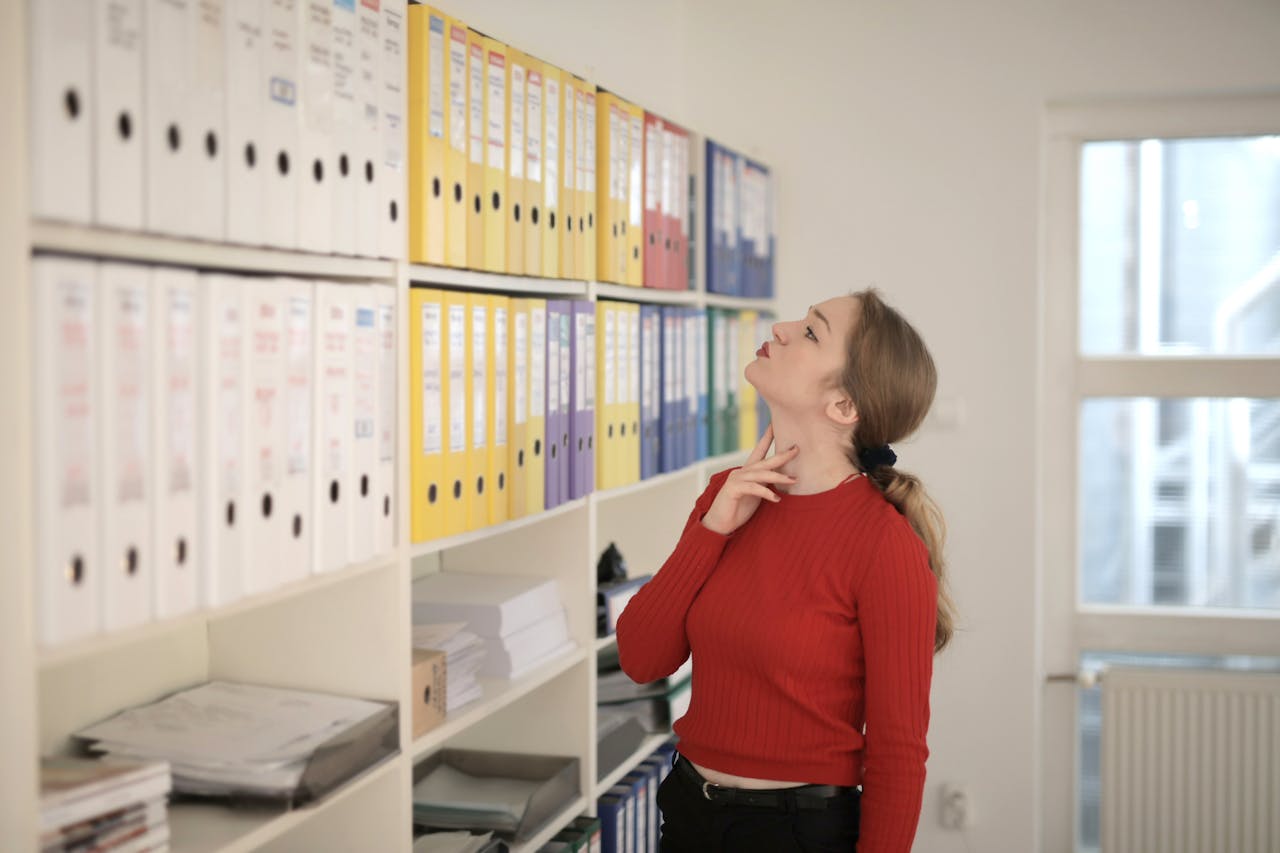
In web development, PHP file handling is the process of reading, writing, and manipulating data that is stored in a file. In PHP, which is a scripting language for server-side applications, file handling is one of the most important aspects of web development.
Table of Contents
In PHP, file handling is used to read configuration files, process user downloads, and store application data.
In this article, you will learn all about the complexities of PHP file handling. We will cover everything from simple operations to complex techniques.
Basics of PHP File Handling Operations:
1. Opening a File
To perform any file operation in PHP, you first need to open the file. The fopen()
function is used for this purpose. It takes two parameters: the file name or path and the mode in which the file will be opened (read, write, append, etc.).
$file = fopen("example.txt", "r");
In this example, we open a file named “example.txt” in read mode ("r"
). The modes include:
"r"
: Read-only."w"
: Write-only. If the file exists, it truncates it; otherwise, it creates a new file."a"
: Write-only. It appends to the file; and creates a new file if it doesn’t exist."x"
: Write-only. Creates a new file; returnsfalse
if the file already exists.
2. Reading from a File
Once a file is opened, you can read its content using functions like fgets()
or fread()
.
Using fgets() to Read a Line
$file = fopen("example.txt", "r"); // Read a line $line = fgets($file); echo $line; fclose($file);
This code reads a single line from “example.txt” and echoes it. The fgets()
function advances the file pointer to the next line.
Using fread() to Read the Entire File
$file = fopen("example.txt", "r"); // Read the entire file $content = fread($file, filesize("example.txt")); echo $content; fclose($file);
Here, fread()
is used to read the entire content of the file. It takes the file handle and the number of bytes to read.
3. Writing to a File
To write content to a file, open it in write or append mode ("w"
or "a"
). Use functions like fwrite()
to add content.
$file = fopen("example.txt", "w"); // Write a line fwrite($file, "Hello, PHP File Handling!"); fclose($file);
In this example, the file is opened in write mode and the fwrite()
the function writes the specified content to the file.
4. Closing a File
It is crucial to close a file after performing operations on it. The fclose()
function is used for this purpose.
$file = fopen("example.txt", "r"); // File operations... fclose($file);
This ensures that the file resources are released and available for other processes.
Advanced PHP File Handling:
1. Checking File Existence
To check if a file exists, use the file_exists()
PHP file handling function.
$file = "example.txt"; if (file_exists($file)) { echo "The file $file exists."; } else { echo "The file $file does not exist."; }
This basic check can help avoid errors when operating on a non-existent file.
Returns true if the file or directory specified by filename exists; false otherwise.
2. File Size and Modification Time
Retrieve information about a file, such as its size and last modification time, using filesize()
and filemtime()
PHP file handling functions.
$file = "example.txt"; echo "File size: " . filesize($file) . " bytes"; echo "Last modified: " . date("F d Y H:i:s.", filemtime($file));
These functions provide insights into the file’s characteristics, aiding in file management tasks.
3. Reading and Writing CSV Files
PHP file handling simplifies reading and writing CSV files with functions like fgetcsv()
and fputcsv()
.
Reading CSV
$file = fopen("data.csv", "r"); while (($data = fgetcsv($file)) !== false) { // Process each row print_r($data); } fclose($file);
This example reads a CSV file line by line and processes each row. The fgetcsv()
function parses the CSV line into an array.
Writing CSV
$file = fopen("output.csv", "w"); $data = array("Name", "Age", "Country"); fputcsv($file, $data); fclose($file);
Here, the fputcsv()
function writes an array of data into the CSV file.
4. Deleting a File
To delete a file, use the unlink()
function.
$file = "example.txt"; if (unlink($file)) { echo "File $file has been deleted."; } else { echo "Unable to delete $file."; }
This simple operation removes a file from the file system.
Deletes filename. Similar to the Unix C unlink() function. An E_WARNING level error will be generated on failure.
5. Checking File Existence and Permissions:
Learn how to use functions like file_exists()
to check if a file exists and is_readable()
/is_writable()
to verify permissions.
$file = "example.txt"; if (file_exists($file) && is_readable($file)) { // Perform operations } else { echo "File does not exist or is not readable."; }
6. Manipulating File Pointers:
Understanding and manipulating file pointers using PHP file handling functions like fseek()
and rewind()
for random access within a file.
$handle = fopen("example.txt", "r"); fseek($handle, 10); // Move pointer to the 10th byte echo fread($handle, 5); // Read 5 bytes from the current position fclose($handle);
7. File Locking:
When multiple processes or scripts may access a file simultaneously, file locking prevents conflicts. The flock()
function can be used for this purpose.
Prevent race conditions and conflicts by implementing file locking using functions like flock()
.
$handle = fopen("example.txt", "a"); if (flock($handle, LOCK_EX)) { // Exclusive lock acquired, perform operations flock($handle, LOCK_UN); // Release the lock } else { echo "Could not acquire lock."; } fclose($handle);
8. Directory Manipulation
PHP enables developers to navigate and manipulate directories with ease. The scandir()
PHP file handling function lists all files and directories in a given path.
$directory = "/path/to/directory"; $contents = scandir($directory); foreach ($contents as $item) { echo $item . "<br>"; }
This example retrieves and displays all items in the specified directory. You can further filter the results based on file types or other criteria.
9. File Streams and Context Options
PHP allows for fine-tuned control over file operations through stream contexts. This is particularly useful when working with remote files or manipulating stream-specific options.
$remoteFile = "https://example.com/somefile.txt"; $options = [ 'http' => [ 'method' => 'GET', 'header' => 'User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64)', ], ]; $context = stream_context_create($options); $remoteContent = file_get_contents($remoteFile, false, $context); echo $remoteContent;
In this example, a stream context is created to set an HTTP header before retrieving the contents of a remote file using file_get_contents()
.
This PHP file handling function is similar to file(), except that file_get_contents() returns the file in a string, starting at the specified offset up to length bytes. On failure, file_get_contents() will return false.
10. Handling File Uploads
Handle file uploads using the $_FILES
superglobal.
$targetDirectory = "uploads/"; $targetFile = $targetDirectory . basename($_FILES["fileToUpload"]["name"]); $uploadSuccess = true; // Check for file existence, size, allowed extensions, etc. if (!$uploadSuccess) { echo "Sorry, your file was not uploaded."; } else { if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $targetFile)) { echo "The file " . basename($_FILES["fileToUpload"]["name"]) . " has been uploaded."; } else { echo "Sorry, there was an error uploading your file."; } }
This example demonstrates a simple file upload form and the corresponding PHP script to handle the file upload.
11. Creating a Directory
Use mkdir()
to create a new directory.
mkdir("new_directory");
12. Deleting a Directory
Use rmdir()
PHP file handling function to remove an empty directory. For recursive deletion, a custom function can be used.
function deleteDirectory($dir) { if (!file_exists($dir)) { return true; } if (!is_dir($dir)) { return unlink($dir); } foreach (scandir($dir) as $item) { if ($item == '.' || $item == '..') { continue; } if (!deleteDirectory($dir . DIRECTORY_SEPARATOR . $item)) { return false; } } return rmdir($dir); } deleteDirectory("directory_to_remove_recursively");
Attempts to remove the directory named by directory. The directory must be empty, and the relevant permissions must permit this. An E_WARNING level error will be generated on failure.
Reference:
Credits:
- Photo by Andrea Piacquadio: https://www.pexels.com/photo/pensive-female-worker-choosing-folder-with-documents-in-modern-office-3791185/
Conclusion:
Learning PHP File Handling is one of the most important skills for every web developer. From user uploads to configuration files, and application data storage to more complex file operations, knowing how to handle files is essential for building strong web applications.
Learn how to handle both simple and complex file operations in PHP, and you’ll be able to handle various file operations in your projects.
Experimenting and testing these operations in various situations will help PHP developers create powerful, feature-packed web applications that efficiently handle files and directories.
Regular practice and testing of these PHP file handling methods will improve your skills and help you solve a wide range of problems in web development.