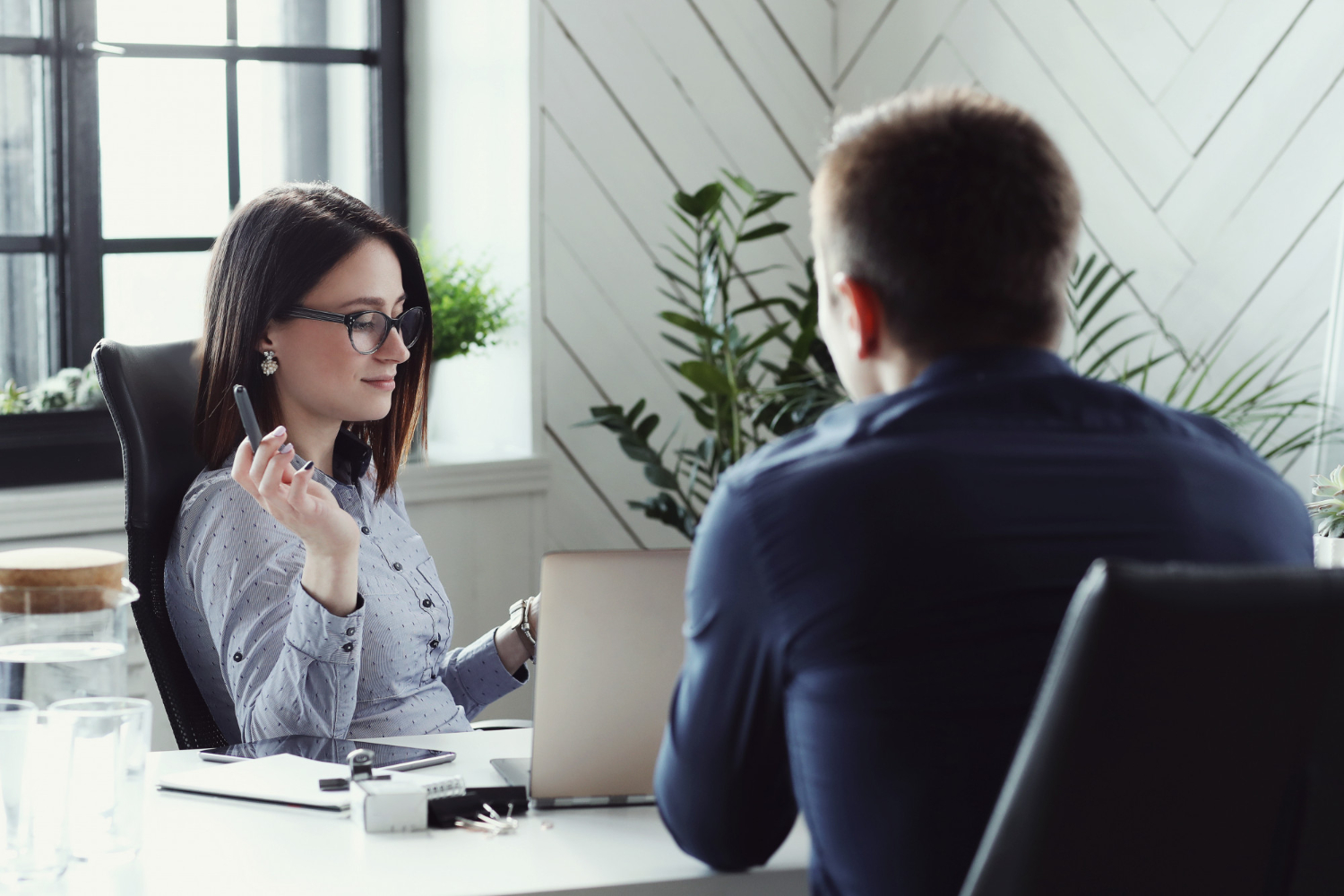
Here is a list of commonly asked CakePHP interview questions that can help you prepare effectively. As a developer preparing for a CakePHP interview, having a clear understanding of its core components, conventions, and features is crucial.
CakePHP is an open-source web framework built on PHP that uses the MVC (Model-View-Controller) architecture. It simplifies development, reduces repetitive coding, and provides powerful functionalities like built-in validation and ORM (Object-Relational Mapping).
List of CakePHP Interview Questions
This comprehensive list of CakePHP Interview Questions covers topics that will help you excel, whether you’re a beginner or an experienced developer.
Bake is a code generation tool included with CakePHP. It automates the creation of Models, Views, Controllers, and test cases. Developers use it to quickly generate the scaffolding of an application. Bake helps reduce the time spent on repetitive tasks:
- To use Bake, run bin/cake bake from the terminal.
hese CakePHP Interview Questions highlight Bake’s importance in automating repetitive tasks:
Helpers in CakePHP are used to simplify the process of writing views. They contain logic for rendering HTML, form elements, and other UI components. Commonly used helpers include:
- HtmlHelper: For generating HTML.
- FormHelper: For creating forms.
- PaginatorHelper: For paginating records.
CakePHP Interview Questions on this topic will check your familiarity with generating dynamic content.
Behaviors are packages of model logic that can be reused across multiple models. They extend the functionality of models. CakePHP Interview Questions about behaviors may cover examples like TimestampBehavior, which automatically handles created and modified fields:
$this->addBehavior('Timestamp');
CakePHP has built-in caching mechanisms to improve performance. Caching can be configured in config/app.php. Common caching engines include:
- FileCache: Stores cached data in files.
- Memcached: Uses Memcached servers.
- RedisCache: Integrates with Redis.
Routing in CakePHP maps URLs to controllers and actions. This allows developers to create user-friendly URLs and handle URL patterns. Routing is configured in config/routes.php
:
$routes->connect('/articles/:id', ['controller' => 'Articles', 'action' => 'view']) ->setPass(['id']) ->setPatterns(['id' => '\d+']);
Associations are used to define relationships between tables in CakePHP. The framework supports the following types:
- BelongsTo: A record in one table belongs to a record in another.
- HasOne: A record in one table has one related record in another.
- HasMany: A record in one table is associated with multiple records in another.
- BelongsToMany: A many-to-many relationship between tables.
$this->belongsTo('Authors'); $this->hasMany('Comments');
Components are reusable packages of logic that are shared between controllers. They provide features like session handling, authentication, and security. In CakePHP Interview Questions, be ready to discuss components like:
- AuthComponent: Handles authentication.
- FlashComponent: Manages flash messages.
- SecurityComponent: Provides security features for handling form tampering and CSRF.
MVC stands for Model-View-Controller. It is a software design pattern used to separate application logic into three interconnected components:
- Model: Manages data logic and business rules (e.g., database access).
- View: Responsible for presenting data to the user (e.g., HTML pages).
- Controller: Handles user input and interaction, updating models and views as necessary.
- beforeFilter(): Runs before each controller action. Useful for authentication checks.
- beforeRender(): Runs before the view is rendered. Often used to set view variables.
CakePHP Interview Questions about these methods focus on their role in request processing.
CakePHP supports associations like BelongsTo, HasOne, HasMany, and BelongsToMany. CakePHP Interview Questions might require you to provide examples of setting up these relationships:
$this->hasMany('Comments'); $this->belongsTo('Authors');
CakePHP uses Object-Relational Mapping (ORM) to interact with the database using objects. CakePHP Interview Questions about ORM will test your understanding of its advantages, like security and ease of use.
CakePHP provides internationalization features using translation files. In CakePHP Interview Questions, be prepared to discuss how the __() function translates strings:
echo __('Welcome to our website');
Fixtures provide sample data for testing purposes. CakePHP Interview Questions on testing might require you to explain how fixtures maintain a consistent database state across test cases.
Middleware modifies the request and response objects. In CakePHP Interview Questions, you may be asked how middleware is configured in src/Application.php
:
$middleware->add(new SecurityMiddleware());
CakePHP uses the principle of Convention over Configuration, which means the framework has a set of conventions to reduce configuration requirements. In CakePHP Interview Questions, understanding this concept is crucial, as it streamlines development and makes code more predictable.
The CakePHP folder structure is essential knowledge for CakePHP Interview Questions. It is organized as:
- app/: Application logic.
- config/: Configuration files.
- logs/: Logs.
- tmp/: Temporary files.
- vendor/: Third-party libraries.
Credits
Conclusion
Preparing for a CakePHP interview questions involves understanding the framework’s structure, features, and how to work with its various components. By reviewing these common questions, you can gain confidence in your knowledge and be better equipped to impress in your interview. Good luck, and happy coding!