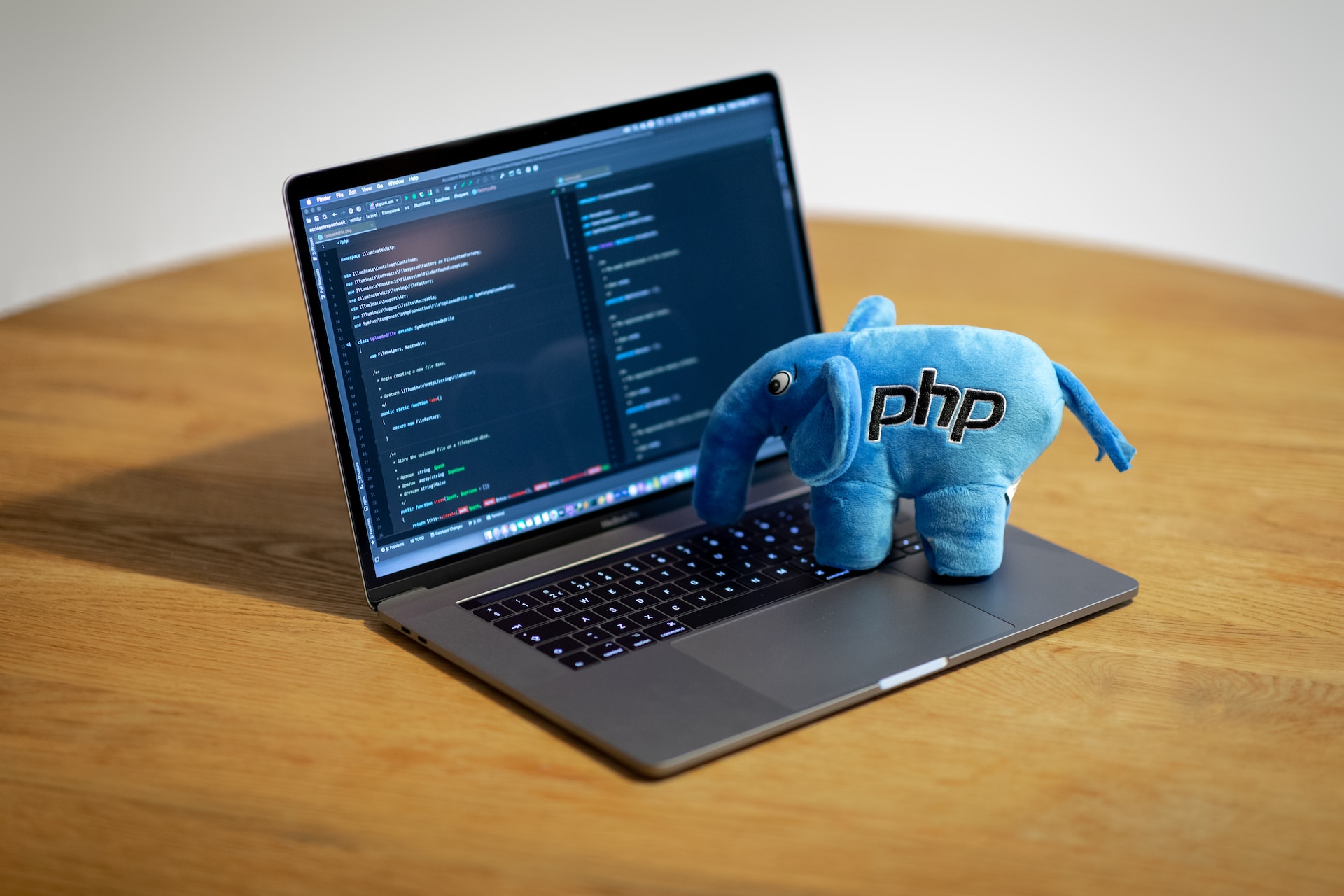
In this article, we’ll learn about PHP Operator.
Table of Contents
In the vibrant orchestra of programming languages, PHP stands as a conductor, orchestrating the beautiful symphony of code.
At the heart of this symphony lies the PHP operators, a collection of melodic elements that harmonize data manipulation, comparisons, and control flow.
We will embark on a musical journey, exploring the exquisite world of PHP operators and how they compose the symphony of code with elegance and precision.
What are PHP Operators
Assignment Operator
The assignment operator is so common in PHP expressions. It’s the equal sign (=) that is used to assign a value to a variable.
$result = 25; // Value of $result is now 25 $result = 'Welcome'; // Value of $result is now Welcome
Arithmetic Operators
Arithmetic operators are for doing mathematical operations on numeric values. There are five arithmetic operators as Addition(+), Subtraction(-), Multiplication(*), Division(/) and Modulus(%).
/* Addition */ $result = 1 + 24; // $result equals to 25 $a = 2; $b = 5.37; $result = $a + $b; // $result equals to 7.37 /* Subtraction */ $result = $b - $a; // $result equals to 3.37 $result = $a - $b; // $result equals to -3.37 /* Multiplication */ $result = $a * $b; // $result equals to 10.74 /* Division */ $result = $b / $a; // $result equals to 2.685
Modulus Operator
The modulus operator gives the remainder of a division operation as the result.
$a = 2; $c = 15; $result = $c % $a; // $result equals to 1 $result = 10 % $a; // $result equals to 0
Integer Division
In some programming languages, there is an operator called Integer Division which lets you have only the integer part after a division.
For example, if you divide 15 by 2 using Integer Division, you would get 7 instead of 7.5. PHP doesn’t provide a direct operator for this but you can get the same result using integer typecasting.
$a = 2; $c = 15; $result = (int)($c / $a); // $result equals to 7
String Concatenation
String concatenation (also called Dot operator) is used for joining two or more strings together.
$name = 'Robin Jackman'; $welcome = 'Welcome '.$name.'!'; // Welcome Robin Jackman!
Increment and Decrement Operators
Increment and decrement operators are unary operators (means operate on only one value). They increment or decrement value of the given operand by one.
$d = 20; echo ++$d; // 21 echo --$d; // 20
Both these operators can be placed after the operand. In that case, value changing happens after returning the current value.
echo $d++; // Will print 20, value of $d is now 21 echo $d; // Will print 21 echo $d--; // Will print 21, value of $d is now 20 echo $d; // Will print 20
Comparison Operators
Comparison operators are used to comparing two values. The result of a comparison operation is always Boolean (means either true or false). These operators are mainly used in control structures.
Greater Than Operator (>)
The result becomes true if left operand is greater than the right operand, false otherwise.
/* Assume $a and $b hold two integer values */ if ($a > $b) { // Will execute code in this block if $a is greater than $b } else { // Will execute code in this block if $a is not greater than $b }
Less Than Operator (<) Result becomes true if the left operand is less than the right operand, false otherwise. Equal Operator (==) Result becomes true if the left operand is equal to the right operand, false otherwise.
Not Equal Operator (!=) Result becomes true if the left operand is not equal to the right operand, false otherwise. Greater Than or Equal Operator (>=)
The result becomes true if the left operand is greater than or equal to the right operand, false otherwise.
$a = 10; $b = 5; $c = 8; $d = 8; ($a >= $d) // true ($b >= $d) // false ($c >= $d) // true
Less Than or Equal Operator (<=) Result becomes true if left operand is less than or equal to the right operand, false otherwise.
Identical Operator (===) Result becomes true only if both operands hold same value and are of same data type.
1. $a = 5; 2. $b = ‘5’; 3. 4. ($a == $b) // true 5. ($a === $b) // false First operation results true since it only checks the value but second operation becomes false since it checks data type in addition to the value ($a is an integer and $b is a string).
Not Identical Operator (!==) Result becomes true only if both operands don’t hold the same value and are not of the same data type.
OR Operator (||)
The result becomes true if at least one operand is true. Instead of || word OR can be used.
XOR Operator
The result becomes true if only one operand is true but not both.
NOT Operator (!)
NOT operator acts on only one operand and the result becomes true if the operand is false and vice versa.
// Assume $result holds a Boolean value if (!$result) { // Show the error message }
Error Suppression Operator (@)
This operator lets you prevent the PHP interpreter from showing any warning/error to the user. This can be helpful in occasions where you want to carry out the rest of the script even though a possible error occurred.
@sendWelcomeMail();
Think that sendWelcomeMail() is a function that sends a welcome email in a user registration process.
If required email resources are not set properly, this function may show PHP errors to the user and abort executing the rest of the script.
Using @ operator lets you get the user registered and carry out the rest of the process (like showing a welcome message) even in a case emailing failed. However, be careful when you use this operator since it can make debugging harder.
Bitwise Operators
Bitwise operators work on the bits of the given operands and are applicable only for integers.
Unless you are working on a project related to bit manipulation, you are unlikely to use these operations in normal PHP projects.
Backtick Operator
Backtick operator lets you execute shell commands and fetch their output. This operator doesn’t work when PHP Safe Mode is enabled which is the case in most production environments.
More Forms of Assignment Operator
The assignment operator can be combined with String Concatenation (.), Arithmetic Operators (+, -, *, /, %) and Bitwise Operators to do operations on a variable and assign the result to the same variable.
$name = 'Mr. '; $name .= 'Robin Jackman'; echo $name; // Would print Mr. Robin Jackman
.= operator can be helpful when you build complex SQL queries which may be longer than normal line length. This operator would let you split them and make them more readable.
Operator Precedence
Operator Precedence comes into play when you have more than one operation in an expression.
$a = 2; $b = 5; $c = 10; $d = $a+$b*$c; // $d equals to 52
In the above expression, first, 5 will be multiplied by 10, and secondly, 2 will be added to the result of the multiplication since Multiplication (*) has precedence over Addition (+).
When you do more than one operation in a single expression, it’s recommended to use brackets to make the code readable and to force the precedence you want.
In the above example, think that you first wanted to carry out the addition. Then you could force that precedence as below.
$d = ($a+$b)*$c; // $a equals to 70
In the symphony of PHP development, operators take on the roles of musical notes, forming melodies, harmonies, and rhythms.
Understanding the diverse range of PHP operators and their unique contributions enables developers to compose beautiful and elegant code symphonies.
So, embrace the crescendo of arithmetic, the harmony of assignments, the counterpoint of comparisons, the chorus of logic, the percussion of bitwise operations, and the improvisation of custom PHP operators.
Conduct the symphony of code with finesse, and witness your PHP compositions resonate with creativity, efficiency, and the joy of programming.