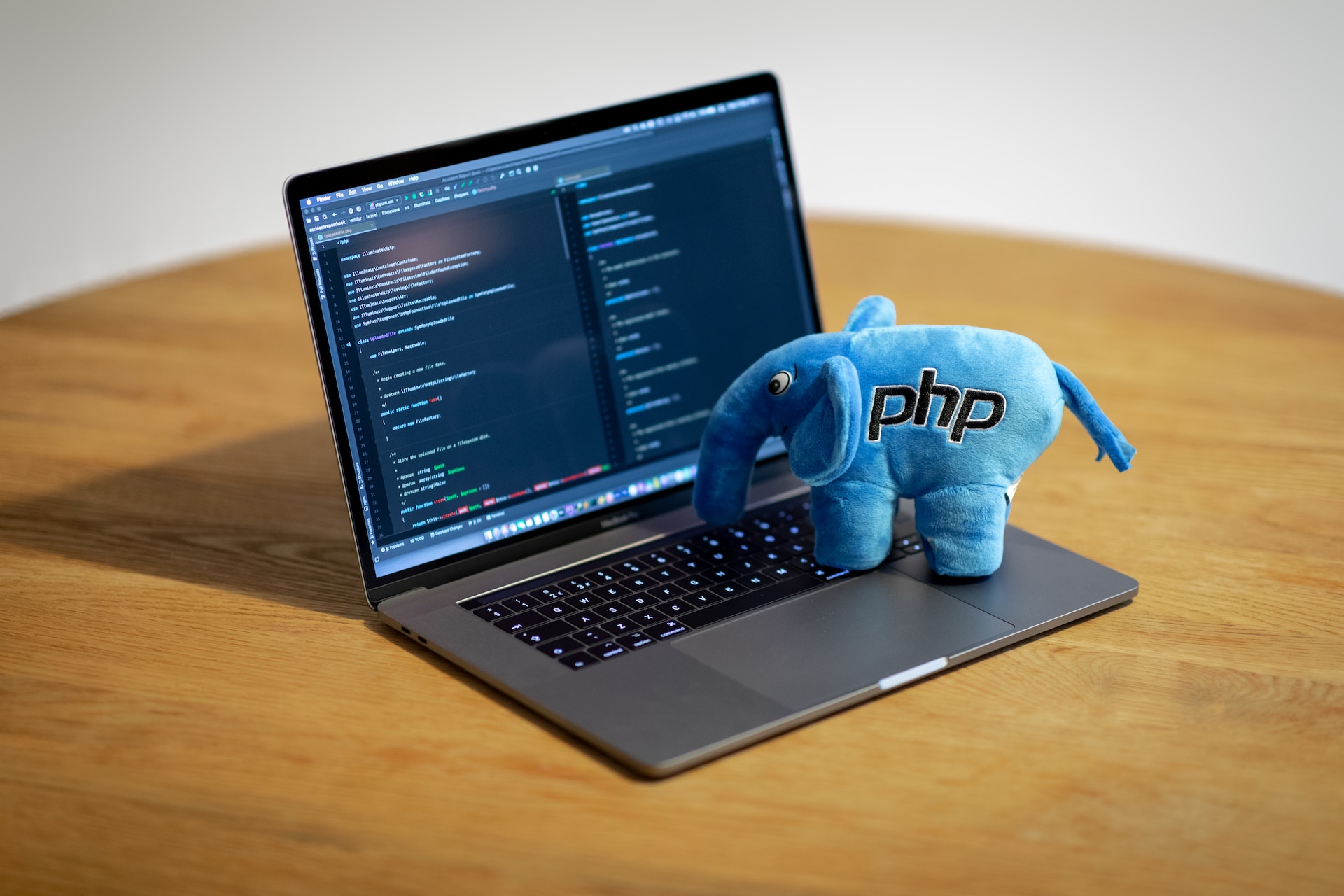
In this article, we’ll see about PHP Filter.
Table of Contents
In the realm of web development, data validation is a critical aspect of ensuring the integrity and security of user inputs. PHP Filter, a powerful built-in extension, provides a comprehensive set of functions for validating and sanitizing various types of data.
We’ll embark on a journey to master PHP Filter, exploring its features, usage scenarios, and best practices to help you build robust and secure web applications.
What is PHP Filter
PHP Filter is used to validate (check the valid format) and sanitize (remove unwanted data) the input data received from an external source. The external source can be a user input, output of a query evaluation, web service data, server variables, cookies, etc.
A filter is a function that can be used to validate and sanitize data. Filters are used to ensure that data is safe to use in a PHP script.
For example, you can use a filter to ensure that a user input is a valid email address. You can also use a filter to ensure that a user input is a valid number.
There are two kinds of filters:
1. Validating filters:
- Are used to validate user input
- Strict format rules (like URL or E-Mail validating)
- Returns the expected type on success or FALSE on failure
2. Sanitizing filters:
- Are used to allow or disallow specified characters in a string
- No data format rules
- Always return the string
PHP Filters
Field | Description |
FILTER_CALLBACK | Call a user-defined function to filter data |
FILTER_SANITIZE_STRING | Strip tags, optionally strip or encode special characters |
FILTER_SANITIZE_STRIPPED | Alias of “string” filter |
FILTER_SANITIZE_ENCODED | URL-encode string, optionally strip or encode special characters |
FILTER_SANITIZE_SPECIAL_CHARS | HTML-escape ‘”<>& and characters with ASCII value less than 32 |
FILTER_SANITIZE_EMAIL | Remove all characters, except letters, digits and !#$%&’*+-/=?^_`{|}~@.[] |
FILTER_SANITIZE_URL | Remove all characters, except letters, digits and $-_.+!*'(),{}|\\^~[]`<>#%”;/?:@&= |
FILTER_SANITIZE_NUMBER_INT | Remove all characters, except digits and +- |
FILTER_SANITIZE_NUMBER_FLOAT | Remove all characters, except digits, +- and optionally .,eE |
FILTER_SANITIZE_MAGIC_QUOTES | Apply addslashes() |
FILTER_UNSAFE_RAW | Do nothing, optionally strip or encode special characters |
FILTER_VALIDATE_INT | Validate value as integer, optionally from the specified range |
FILTER_VALIDATE_BOOLEAN | Return TRUE for “1”, “true”, “on” and “yes”, FALSE for “0”, “false”, “off”, “no”, and “”, NULL otherwise |
FILTER_VALIDATE_FLOAT | Validate value as float |
FILTER_VALIDATE_REGEXP | Validate value against regexp, a Perl-compatible regular expression |
FILTER_VALIDATE_URL | Validate value as URL, optionally with required components |
FILTER_VALIDATE_EMAIL | Validate value as e-mail |
FILTER_VALIDATE_IP | Validate value as IP address, optionally only IPv4 or IPv6 or not from private or reserved ranges |
How to Use Filter:
To use a filter, you first need to know the name of the filter. You can find the names of the available filters in the PHP documentation.
Once you know the name of the filter, you can use it in your PHP code. To do this, you use the filter_var()
function.
The filter_var()
function takes two arguments:
- The first argument is the value that you want to filter.
- The second argument is the name of the filter.
To validate data using filter extension you need to use the PHP’s filter_var()
function. The basic syntax of this function can be given with:
filter_var(variable, filter, options)
The following example will show you how to sanitize and validate an e-mail address.
<?php // Sample email address $email = "someone@@example.com"; // Remove all illegal characters from email $email = filter_var($email, FILTER_SANITIZE_EMAIL); // Validate e-mail address if(filter_var($email, FILTER_VALIDATE_EMAIL)){ echo "The <b>$email</b> is a valid email address"; } else{ echo "The <b>$email</b> is not a valid email address"; } ?>
PHP Filter provides a comprehensive suite of functions for data validation and sanitization, empowering developers to create secure and reliable web applications. By mastering PHP Filter, you can ensure the integrity of user inputs, prevent security vulnerabilities, and deliver a smooth user experience.
This guide has equipped you with the knowledge to effectively validate and sanitize various types of data, customize validation rules, handle file uploads, and cater to internationalization requirements. Armed with this expertise, you’re ready to build robust and secure PHP applications. Happy coding with PHP Filter!
I as well conceive so , perfectly written post! .