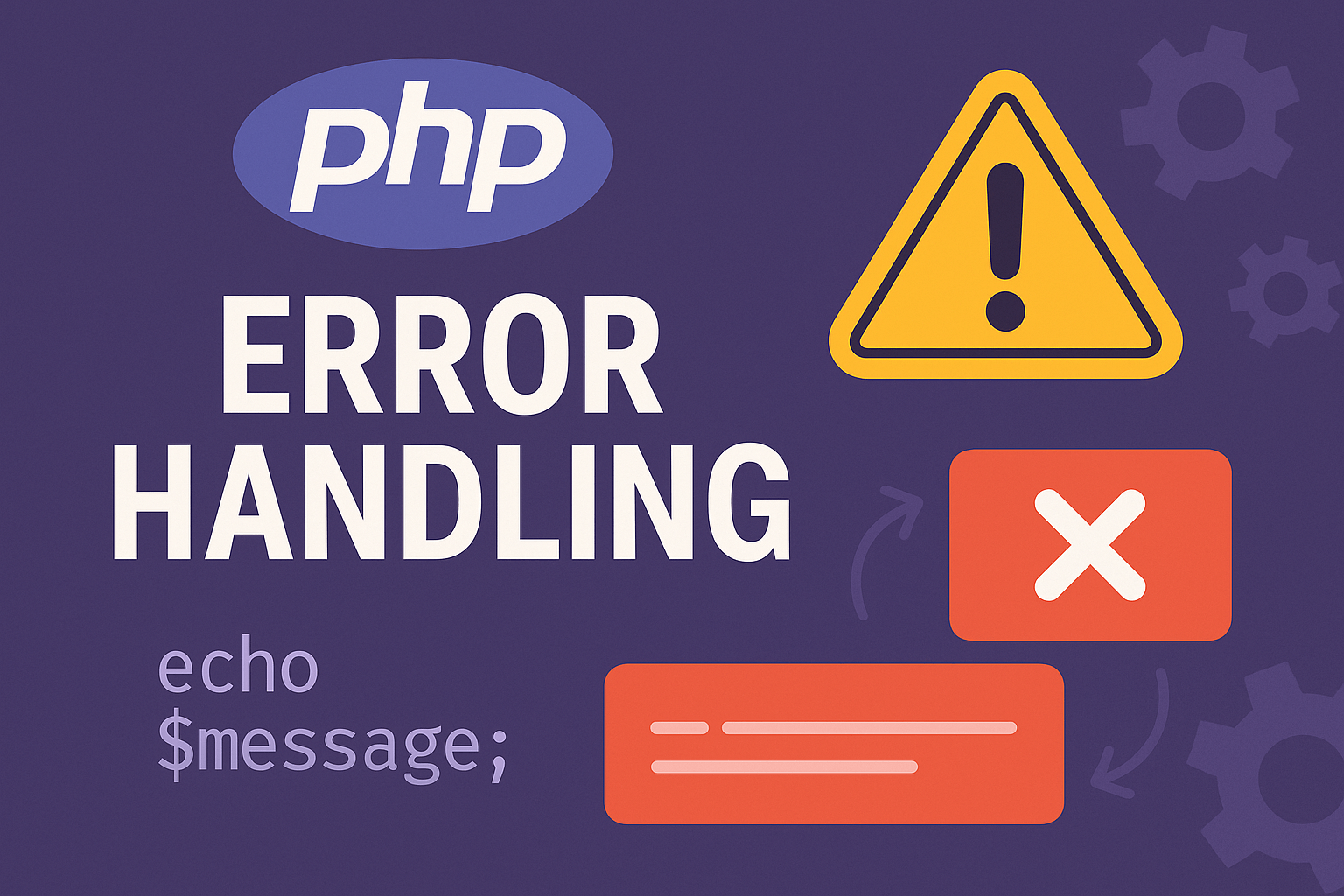
In this article, we will explore PHP error handling and discuss the best practices for handling errors in your PHP applications.
Table of Contents
What is PHP Error Handling
PHP error handling is the process of identifying, reporting, and responding to errors or exceptions that occur during the execution of a PHP script. It involves detecting and handling various types of errors that can occur during the execution of a PHP script, such as syntax errors, runtime errors, and logical errors.
PHP provides several built-in functions and features that developers can use to handle errors, including:
- Error reporting: PHP provides various levels of error reporting, which can be set using the error_reporting() function. These levels include displaying all errors, displaying no errors, or displaying only specific types of errors.
- Exceptions: PHP allows developers to throw and catch exceptions, which are objects that represent errors or exceptional conditions that occur during the execution of a script. Exceptions can be caught and handled using try-catch blocks.
- Error logging: PHP can log errors to a file or system log using the error_log() function. This allows developers to review and troubleshoot errors that occur during runtime.
- Custom PHP Error Handling: PHP allows developers to define their own functions using the set_error_handler() function. This can be useful for PHP Error Handling in a custom way, such as sending an email or displaying a custom error page.
- Handling errors in code using conditional statements or die() function
Understanding PHP Errors
PHP errors are typically categorized into three types: notices, warnings, and fatal errors.
Notices are non-critical errors that do not prevent the script from executing. For example, a notice might be issued if you try to use an undefined variable.
Warnings are more serious than notices and indicate that there is a potential problem with the script. For example, a warning might be issued if you try to open a file that does not exist.
Fatal errors are the most serious error and cause the script to terminate immediately. For example, a fatal error might be issued if you try to call a function that does not exist.
PHP Error Reporting
Error reporting in PHP is a built-in feature that allows developers to identify and display errors that occur during the execution of a PHP script. By default, error reporting in PHP is set to display all errors except for notices and strict warnings.
To customize the error reporting level in PHP, developers can use the error_reporting() function. This function takes a single argument, which is a bitmask that specifies which errors should be reported.
Here are some examples of how to use the error_reporting() function:
To display all errors, including notices and strict warnings:
error_reporting(E_ALL);
Display all errors except notices and strict warnings:
error_reporting(E_ALL & ~E_NOTICE & ~E_STRICT);
and to display only fatal errors:
error_reporting(E_ERROR);
In addition to setting the error reporting level, PHP also provides a function called ini_set() that can be used to change the value of the error_log directive. This allows developers to redirect error messages to a file instead of displaying them on the screen. For example, to log errors to a file called “error_log.txt”, you can use the following code:
ini_set('error_log', 'error_log.txt');
By default, error reporting in PHP is turned on, but it can be disabled by setting the display_errors directive to Off in the php.ini file. However, it is recommended that error reporting be enabled during development and testing to catch and fix errors early on.
Following are the values that we can provide for setting various error levels:
Reporting Level | Description |
---|---|
E_WARNING | Only displays warning messages and doesn’t stop the execution of the script. |
E_NOTICE | Displays notice that occurs during normal code execution. |
E_USER_ERROR | Display user-generated errors i.e the custom error handlers. |
E_USER_WARNING | Display user-generated warning messages. |
E_USER_NOTICE | Display user-generated notices. |
E_RECOVERABLE_ERROR | Displays non-fatal errors. |
E_ALL | Display all errors and warnings. |
PHP Exception Handling
Another approach to PHP Error Handling is to use exception handling. Exceptions are a powerful way to handle errors because they allow you to separate the error-handling logic from the rest of your application.
Exceptions are a way of signaling that an error has occurred and allowing code to be executed to handle the error, rather than simply terminating the script or outputting an error message.
Like all other programming languages, PHP also has an exception-handling model. There are three keywords related to exception handling in PHP. These are as follows:
Keyword | Description |
---|---|
try | In the “try” block, codes are written in which there may be an exception. If there is no exception, this code execution will continue. Otherwise, it “throws” an exception. |
throw | If an exception is triggered, the control is transferred from the try block to the catch block using the keyword “throw”. Each “throw” must have a “catch”. |
catch | The catch block catches the exception and creates an object which will hold exception information. |
To throw an exception in PHP, you can use the throw statement followed by an instance of the Exception class or a subclass of Exception. For example:
function divide($numerator, $denominator) { if ($denominator == 0) { throw new Exception("Cannot divide by zero"); } return $numerator / $denominator; } try { $result = divide(10, 0); echo "Result: $result"; } catch (Exception $e) { echo "Caught exception: " . $e->getMessage(); }
In this example, the divide() function checks if the denominator is zero and throws an exception with an error message if it is. The try block contains the code that may throw an exception, and the catch block contains the code that will handle the exception if it is thrown. If an exception is thrown, control is transferred to the catch block and the error message is displayed.
You can also define your own custom exception classes by extending the Exception class. For example:
class DivideByZeroException extends Exception {} function divide($numerator, $denominator) { if ($denominator == 0) { throw new DivideByZeroException("Cannot divide by zero"); } return $numerator / $denominator; } try { $result = divide(10, 0); echo "Result: $result"; } catch (DivideByZeroException $e) { echo "Caught exception: " . $e->getMessage(); } catch (Exception $e) { echo "Caught general exception: " . $e->getMessage(); }
In this example, a custom DivideByZeroException class is defined, which extends the Exception class. The divide() function throws an instance of this exception if the denominator is zero. The catch block for DivideByZeroException is executed if that exception is thrown, and the catch block for Exception is executed if any other exception is thrown.
Following are some of the functions that can be used:
- getMessage() − Prints exception message.
- getCode() −Prints exception code.
- getFile() − Prints source filename.
- getLine() − Prints source line.
- getTrace() − Prints n-array of the backtrace().
- getTraceAsString() − Prints the formatted string of trace.
Error logging
PHP error logging is a mechanism for recording errors and warnings that occur during the execution of a PHP script to a log file, instead of displaying them on the screen.
This is useful for tracking down and fixing errors that occur on a live website or application.
PHP provides several functions and directives for configuring error logging:
- error_reporting: This function sets the level of error reporting for the script. By default, all errors are reported except for notices and strict warnings. The recommended setting for error reporting during development is E_ALL, which reports all errors.
- ini_set: This function sets the value of a configuration directive at runtime. The error_log directive controls where error messages are logged. By default, error messages are logged to the web server’s error log, but you can specify a custom log file by setting the error_log directive.
Here’s an example of how to configure error logging in PHP:
// Enable error reporting and log all errors to a file error_reporting(E_ALL); ini_set('log_errors', 1); ini_set('error_log', '/path/to/error_log.txt');
This code sets the error reporting level to E_ALL, which reports all errors and enables error logging. The log_errors directive is set to 1 to enable logging, and the error_log directive is set to the path of the log file where errors should be written.
Once error logging is enabled, PHP will write error messages to the specified log file, which can be viewed using a text editor or log viewer tool. The log file will contain a record of each error that occurs, along with a timestamp, the error message, and other relevant information.
By logging errors in PHP, you can diagnose and fix issues with your code more quickly and easily, and ensure that your website or application is running smoothly for your users.
Custom PHP Error Handling
Custom PHP Error Handling allows you to handle errors and exceptions in a specific way for your application. This can be useful if you need to customize error messages, log errors to a database or external service, or take other actions when an error occurs.
To implement custom PHP Error Handling, you can define a function that will be called whenever an error occurs, using the set_error_handler() function. Here’s an example:
function myErrorHandler($errno, $errstr, $errfile, $errline) { // Log the error to a file or external service error_log("Error $errno: $errstr in $errfile on line $errline"); // Display a custom error message to the user echo "An error occurred. Please try again later."; // Stop processing the script exit; } // Set the custom error handler set_error_handler("myErrorHandler");
In this example, the myErrorHandler() function is defined to log errors to a file or external service, display a custom error message to the user, and stop processing the script. The set_error_handler() function is then called to set this function as the custom error handler.
You can also define a function to handle exceptions using the set_exception_handler() function. Here’s an example:
function myExceptionHandler($exception) { // Log the exception to a file or external service error_log("Exception: " . $exception->getMessage()); // Display a custom error message to the user echo "An error occurred. Please try again later."; // Stop processing the script exit; } // Set the custom exception handler set_exception_handler("myExceptionHandler");
In this example, the myExceptionHandler() function is defined to log exceptions to a file or external service, display a custom error message to the user, and stop processing the script. The set_exception_handler() function is then called to set this function as the custom exception handler.
Using die() Function
The die() method terminates the execution of the current PHP script and prints a message as output.
In PHP, the die() function is used to immediately terminate the script execution and display an error message. It is often used for PHP Error Handling and debugging purposes.
For example, if you want to display an error message and stop the script execution if a file cannot be opened, you can use the die()
function as follows:
$file = fopen("example.txt", "r") or die("Unable to open file!");
If the file cannot be opened, the die() function will be called, displaying the error message “Unable to open file!” and stopping the script execution.
However, using die() to handle errors is generally not considered to be the best practice in PHP development. Instead, it is recommended to use try-catch blocks and custom exception handling to gracefully handle errors and provide more meaningful error messages to users.
PHP error handling is an essential aspect of developing secure and reliable web applications. By understanding the different types of errors and implementing best practices for PHP Error Handling, you can ensure that your PHP applications are robust and performant. I hope this article helps you to learn about PHP Error Handling!