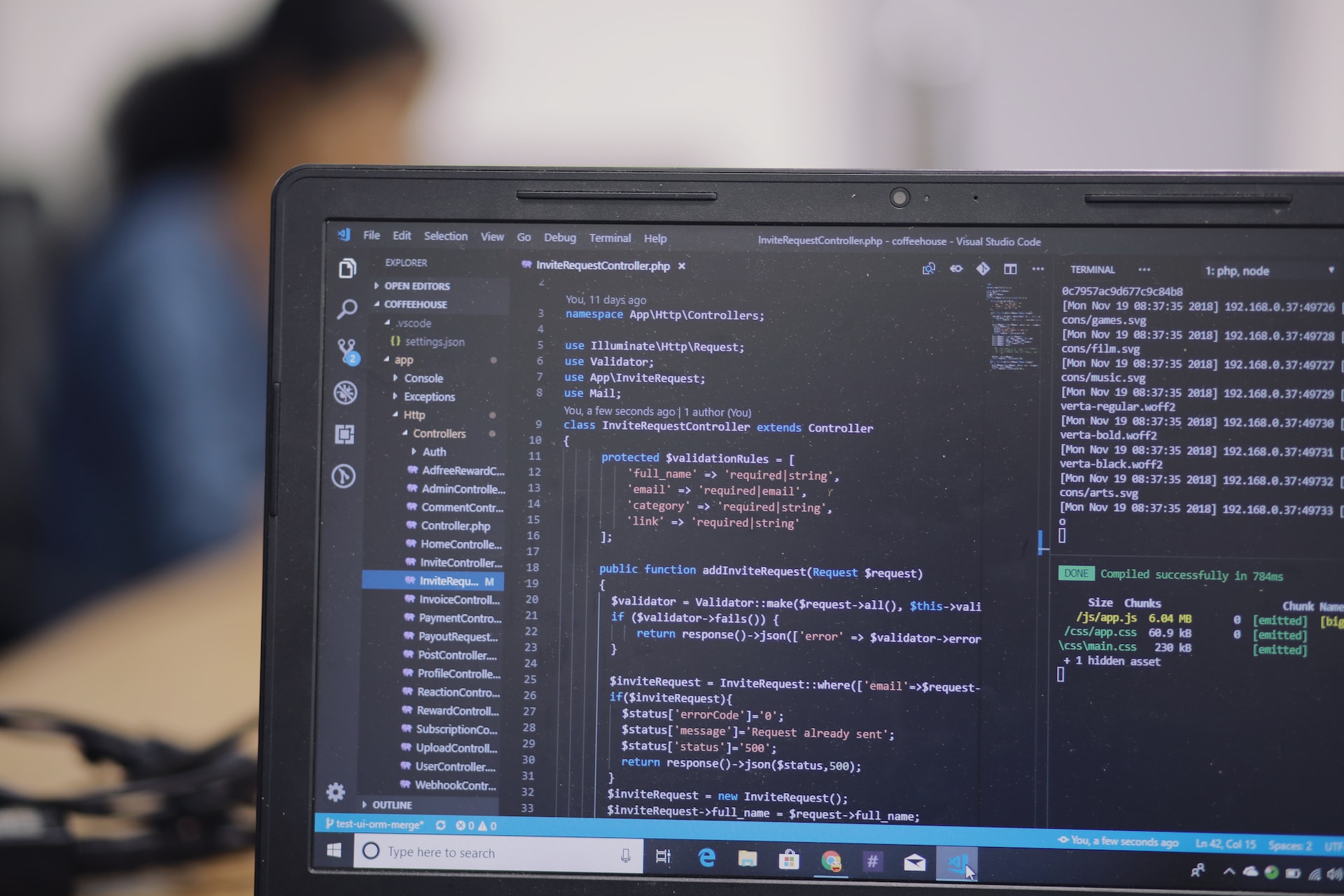
In this article, we’ll learn about PHP Abstract Class and Interface.
Table of Contents
Welcome to the exciting world of PHP programming! We’ll delve into the powerful tools that php abstract class and interfaces offer to PHP developers like yourself.
These concepts may sound daunting at first, but fear not! We’re here to demystify them and show you how they can make your code more organized, reusable, and flexible.
Whether you’re a seasoned developer or just starting your PHP journey, this article will equip you with the knowledge to wield php abstract class and interfaces effectively and take your coding skills to the next level.
PHP Abstract Class and Interface:
PHP Abstract class and interfaces are two important concepts in object-oriented programming (OOP) in PHP. Abstract classes provide a way to define common functionality that can be shared by multiple classes, while interfaces define a contract that must be implemented by any class that claims to implement it.
Abstract class
Abstraction is a way of hiding information.
In abstraction, there should be at least one method that must be declared but not defined.
The class that inherits this abstract class needs to define that method.
There must be an abstract keyword that must be returned before this class for it to be an abstract class.
This class cannot be instantiated. only the class that implements the methods of abstract class can be instantiated.
There can be more than one method that can be left undefined.
<?php abstract class a { abstract function b(); public function c() { echo "Can be used as it is"; } } class m extends a { public function b() { echo "Defined function b<br/>"; } } $tClass = new m(); $tClass->b(); $tClass->c(); ?>
Defined function b
Can be used as it is
in the above example class a is a abstract class and it contains a abstract method b().
the child class m inherit class a in which abstract method be is defined completely.
<?php abstract class A { abstract function f1(); function f2() { echo "hello"; } } class B extends A { function f1() { echo "hi"; } } $ob=new B(); $ob->f1(); ?>
hi
Interface
The class that is fully abstract is called an interface.
Any class that implements this interface must use the implements keyword and all the methods that are declared in the class must be defined here. otherwise, this class also needs to be defined as abstract.
Multiple inheritances is possible only in the case of the interface.
<?php interface A { function f1(); } interface B { function f2(); } class C implements A,B { function f1() { echo "hi"; } function f2() { echo "hello"; } } $ob=new C(); $ob->f1(); $ob->f2(); ?>
hello
in the above example there are two interfaces A and B. a class c implements both interfaces and define the methods f1() and f2()
of interfaces A and B respectively. if any of the methods of interfaces are left undefined in the class that implements the interface then
it must be defined as abstract.
When to Use PHP Abstract Class and Interface
PHP Abstract class and interfaces are both powerful tools that can be used to improve the design and implementation of OOP code in PHP. However, it is important to use them correctly.
Abstract classes should be used when there is a set of common functionality that can be shared by multiple classes. Interfaces should be used when you want to ensure that classes have a certain set of functionality, but you don’t want to specify how that functionality is implemented.
In general, abstract classes should be used for larger, more complex pieces of functionality, while interfaces should be used for smaller, more discrete pieces of functionality.
By using PHP abstract class and interfaces correctly, you can improve the design and implementation of your PHP code, making it easier to read, maintain, and test.
Congratulations! You’ve unlocked the magic of abstract classes and interfaces in PHP. These powerful tools will transform the way you write code, making it more modular, maintainable, and adaptable.
Embrace them with confidence, experiment with different use cases, and watch your PHP projects reach new heights of elegance and efficiency.
Remember, PHP abstract class and interfaces are your allies in crafting exceptional software. Happy coding!
Hope this article helps you to understand object-oriented programming terms in PHP called Abstract Class and Interface