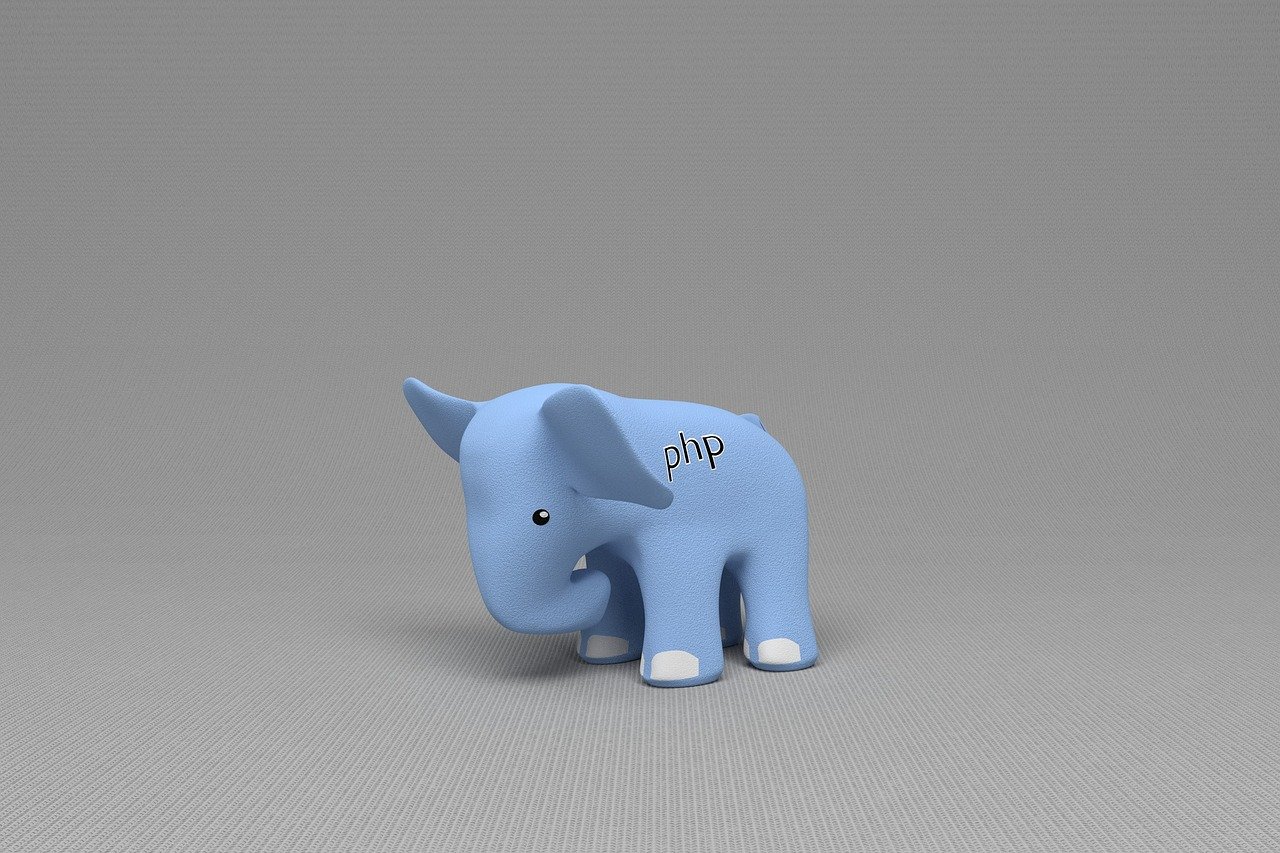
PHP 8.3 was released on November 23, 2023, and has many new features and improvements since the launch of PHP 8.2.
Table of Contents
PHP 8.3 contains many new features, such as explicit typing of class constants, deep-cloning of readonly properties and additions to the randomness functionality. As always it also includes performance improvements, bug fixes, and general cleanup.
PHP, a popular programming language for server-side scripting, keeps advancing with every update, offering a variety of enhancements, fresh functionalities, and better performance.
PHP 8.3, the most recent significant update, is a prime example. This article will explore the thrilling new functionalities and modifications in PHP 8.3, showcasing how they can boost your development process and refine your code.
List of PHP 8.3 Features and Improvements:
Typed Class Constants
Typed class constants enable you to declare a constant with an explicit type within a class. Before PHP 8.3, class constants were untyped, meaning any value could be assigned, potentially leading to type-related errors. Typed class constants help prevent such errors by enforcing a specific type.
Here’s a basic example to illustrate the usage of typed class constants:
class Config { public const int MAX_USERS = 100; public const string APP_NAME = 'MyApplication'; public const float VERSION = 1.0; public const bool DEBUG_MODE = false; }
In this example, MAX_USERS
is explicitly typed as an int
, APP_NAME
as a string
, VERSION
as a float
, and DEBUG_MODE
as a bool
.
json_validate function
PHP 8.3 introduces a fresh capability called json_validate, which determines if the provided string is a valid JSON string by returning a boolean value of true or false.
Applications that accept user-provided JSON, or connect remote JSON APIs may find the best use of the new json_validate
, because there is a significant chance of encountering invalid JSON strings.
Here’s a basic example demonstrating how to use json_validate()
:
$jsonString = '{"name": "John", "age": 30, "city": "New York"}'; if (json_validate($jsonString)) { echo "The JSON string is valid."; } else { echo "The JSON string is invalid."; }
json_validate()
allows to check if a string is syntactically valid JSON, while being more efficient than json_decode()
.
New #[\Override] Attribute
The #[\Override]
attribute in PHP 8.3 is used to explicitly mark methods in a subclass that are intended to override methods in a parent class. This attribute serves as a way to enforce and verify method overriding at the time of development, providing an additional layer of code correctness.
Using the #[\Override]
the attribute is straightforward. You place the attribute above the method definition in the child class that is intended to override a method in the parent class.
Here’s a simple example to illustrate its usage:
class ParentClass { public function displayMessage(): void { echo "Message from ParentClass"; } } class ChildClass extends ParentClass { #[\Override] public function displayMessage(): void { echo "Message from ChildClass"; } } $child = new ChildClass(); $child->displayMessage(); // Output: Message from ChildClass
In this example, the ChildClass method displayMessage is marked with the #[\Override] attribute, indicating that it is intended to override the displayMessage method in ParentClass.
By adding the #[\Override] attribute to a method, PHP will ensure that a method with the same name exists in a parent class or in an implemented interface. Adding the attribute makes it clear that overriding a parent method is intentional and simplifies refactoring, because the removal of an overridden parent method will be detected.
Deep-cloning of readonly properties
Deep-cloning is the process of creating a new object that is a copy of an existing object, along with copies of all objects referenced by the original object.
Readonly properties were introduced in PHP 8.1, allowing developers to define properties that can be initialized once and cannot be modified thereafter. This feature is useful for creating immutable objects, where the state of an object cannot change after it has been constructed.
In PHP 8.3, deep-cloning support for objects with readonly properties has been introduced. This feature allows developers to create deep copies of objects while maintaining the immutability guarantees provided by readonly properties.
readonly
properties may now be modified once within the magic __clone
method to enable deep-cloning of readonly properties.
Consider the following example of a nested object structure with readonly properties:
class Address { public readonly string $city; public readonly string $country; public function __construct(string $city, string $country) { $this->city = $city; $this->country = $country; } } class User { public readonly string $name; public readonly Address $address; public function __construct(string $name, Address $address) { $this->name = $name; $this->address = $address; } } $originalAddress = new Address("New York", "USA"); $originalUser = new User("John Doe", $originalAddress); // Perform a deep clone $clonedUser = clone $originalUser; // Verify deep cloning echo $originalUser->address === $clonedUser->address ? "Shallow Clone" : "Deep Clone"; // Output: Deep Clone
In this example, when $originalUser is cloned, both the User object and the Address object are duplicated. The cloned User object has its own copy of the Address object, ensuring that changes to one do not affect the other.
Dynamic class constant fetch
PHP 8.3 introduces dynamic class constant fetching, which allows developers to access class constants dynamically using variable names. This feature adds flexibility, particularly in scenarios where constant names are determined at runtime. In this blog post, we will explore this feature with practical examples.
Here’s a basic example to illustrate dynamic class constant fetching:
class Foo { const PHP = 'PHP 8.3'; } $searchableConstant = 'PHP'; var_dump(Foo::{$searchableConstant}); // Output: string(6) "PHP 8.3"
In this example:
- The Foo class defines a constant named PHP.
- The variable $searchableConstant holds the name of the constant to fetch.
- Foo::{$searchableConstant} dynamically accesses the PHP constant in the Foo class.
PHP CLI Linting Supports Multiple Files in PHP 8.3
Linting is the process of analyzing source code to identify potential errors and coding standard violations. In PHP, linting primarily focuses on detecting syntax errors using the php -l
(lint) command.
With PHP 8.3, you can pass multiple file paths to the php -l
command, and it will check each file for syntax errors in sequence. This reduces the overhead of running multiple commands and helps quickly identify syntax issues across your codebase.
Suppose you have two PHP files, foo.php
and bar.php
, and you want to check both files for syntax errors. Here’s how you can do it with the enhanced linting feature:
php -l foo.php bar.php
When you run this command, PHP will lint both foo.php
and bar.php
, and output the results for each file:
No syntax errors detected in foo.php No syntax errors detected in bar.php
This new capability allows developers to check multiple PHP files for syntax errors in a single command, improving efficiency and simplifying the development workflow.
New mb_str_pad function
The mb_str_pad() function is designed to pad multibyte strings to a specified length using a specified padding string. This is particularly useful when working with strings that contain characters from non-ASCII character sets, such as emoji or characters from various languages.
The basic syntax of mb_str_pad() is as follows:
string mb_str_pad( string $input, int $pad_length, string $pad_string = " ", int $pad_type = STR_PAD_RIGHT, ?string $encoding = null )
- $input: The input string to be padded.
- $pad_length: The desired length of the resulting string after padding.
- $pad_string: The string to use for padding. Defaults to a space (” “).
- $pad_type: The padding type, which can be STR_PAD_RIGHT (default), STR_PAD_LEFT, or STR_PAD_BOTH.
Let’s look at some practical examples to understand how mb_str_pad()
works.
<?php var_dump(mb_str_pad('▶▶', 6, '❤❓❇', STR_PAD_RIGHT)); // string(18) "▶▶❤❓❇❤" ?>
Here, the input string ‘▶▶’ is padded to the right with ‘❤❓❇’, resulting in ‘▶▶❤❓❇❤’.
Next, we pad the string ‘▶▶’ to a length of 6 using the string ‘❤❓❇’ and left padding.
<?php var_dump(mb_str_pad('▶▶', 6, '❤❓❇', STR_PAD_LEFT)); // string(18) "❤❓❇❤▶▶" ?>
In this case, the input string ‘▶▶’ is padded to the left with ‘❤❓❇’, resulting in ‘❤❓❇❤▶▶’.
New DOMElement::getAttributeNames() Method
The DOMElement::getAttributeNames() method returns an array containing the names of all attributes present on the specified DOM element. This method is particularly useful when you need to iterate over attributes or check for the existence of specific attributes without manually parsing the element.
Let’s explore a practical example to understand how DOMElement::getAttributeNames() works.
<?php $dom = new DOMDocument(); $dom->loadXML('<html xmlns:some="some:ns" some:test="a" test2="b"/>'); $attributeNames = $dom->documentElement->getAttributeNames(); var_dump($attributeNames); ?>
This will output:
array(3) { [0] => string(10) "xmlns:some" [1] => string(9) "some:test" [2] => string(5) "test2" }
Also, there are some methods like DOMElement::insertAdjacentElement()
, DOMElement::insertAdjacentText()
, DOMElement::toggleAttribute()
, DOMNode::contains()
, DOMNode::getRootNode()
, DOMNode::isEqualNode()
, DOMNameSpaceNode::contains()
, and DOMParentNode::replaceChildren()
Credits:
- Image by Aleksey Nemiro from Pixabay
Reference:
Conclusion
PHP 8.3 continues the tradition of enhancing the language with powerful features and optimizations. From Intersection Types and Readonly Classes to improved performance and error handling, PHP 8.3 offers a plethora of tools to make your development process more robust and efficient.
As you explore these new features, you’ll find that PHP 8.3 not only simplifies complex tasks but also empowers you to write cleaner, more maintainable code. Upgrade to PHP 8.3 and take advantage of these cutting-edge improvements in your projects today!