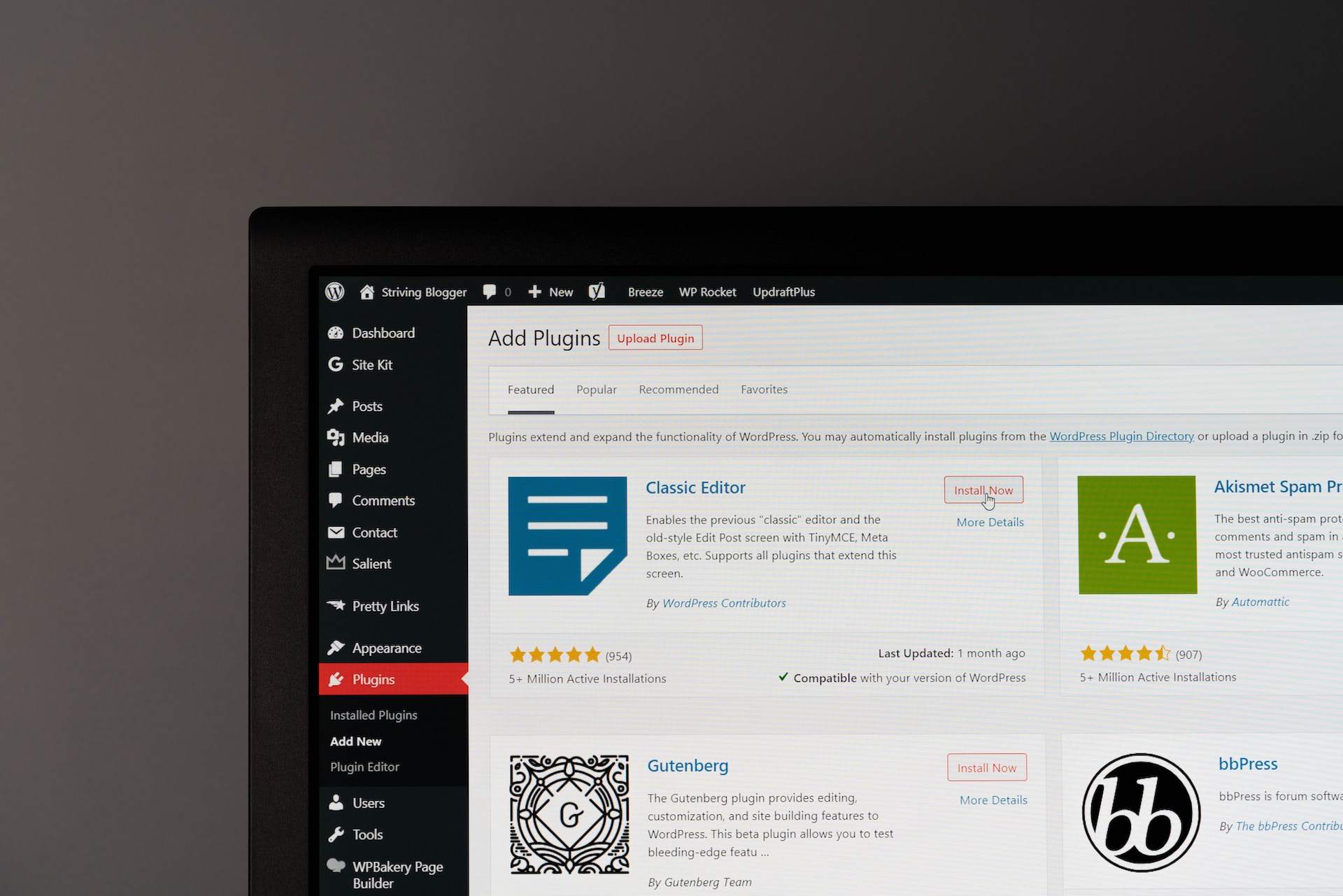
In this article, we’ll see some WordPress Useful Functions
WordPress, the popular content management system (CMS), empowers millions of websites around the world. One of the key reasons for its widespread adoption is the extensive collection of functions and APIs that make development easier and more efficient.
We will dive into the world of WordPress useful functions, exploring a range of powerful tools that can supercharge your development workflow. From enhancing site functionality to streamlining code, these functions will become invaluable assets in your WordPress arsenal.
WordPress Useful Functions
The followings are a list of WordPress Helpful Functions for developers:
1 . Basic Pagination
By default WordPress displays previous/next links at the end of your post list. This can actually be done with a little work using the paginate_links()
functions. The following example from the codex shows how you can add it to a default loop but adding it to custom loops is not much of a stretch.
global $wp_query;
$big = 999999999; // need an unlikely integer
echo paginate_links( array(
'base' => str_replace( $big, '%#%', esc_url( get_pagenum_link( $big ) ) ),
'format' => '?paged=%#%',
'current' => max( 1, get_query_var('paged') ),
'total' => $wp_query->max_num_pages
) );
2 . Custom Block Type For Gutenberg Editor:
If you are using new wordpress editor and want to user custom block then add following code:
register_block_type( 'my-plugin/new-block', array(
'title' => 'New Block',
'icon' => 'megaphone',
'category' => 'widgets',
'render_callback' => 'render_function_callback',
) );
3 . wp_die()
You can use this function to stop PHP execution. You can add the message, title, and additional arguments to be displayed, for example:
wp_die( "Sorry, you don't have access to the", "Permission Denied" );
4 . wp_redirect()
The WordPress redirection function allows you to set a URL to redirect to, and also set a status code, great for handling permanent redirects as well.
wp_redirect( 'http://website.com/your-url/', 301 );
5 . wp_is_mobile()
This function detects when a user is on a mobile device and allows you to display content accordingly. For example:
<?php if( wp_is_mobile() ) : ?>
This is mobile only content are
<?php endif ?>
6 . Remove WordPress Version Number
It is one of WordPress useful functions for remove wordpress version number. If you want to remove the WordPress version number from your site. Simply add this code to your functions file.
function wpb_remove_version() {
return '';
}
add_filter('the_generator', 'wpb_remove_version');
7 . Create Custom WordPress Dashboard Widget
If you want to show custom widget on admin dashboard page, add following code to functions.php file
/**
* Create custom WordPress dashboard widget
*/
function dashboard_widget_function() {
echo '
<h2>Custom Dashboard Widget</h2>
<p>Custom content here</p>
';
}
function add_dashboard_widgets() {
wp_add_dashboard_widget( 'custom_dashboard_widget', 'Custom Dashoard Widget', 'dashboard_widget_function' );
}
add_action( 'wp_dashboard_setup', 'add_dashboard_widgets' );
8 . Remove All Dashboard Widgets
It is one of WordPress useful functions for remove all dashboard widgets .If you want to hide add all default widgets on admin dashboard page, add following code to functions.php file:
/* Remove all dashboard widgets */
function remove_dashboard_widgets() {
global $wp_meta_boxes;
unset( $wp_meta_boxes['dashboard']['side']['core']['dashboard_quick_press'] );
unset( $wp_meta_boxes['dashboard']['normal']['core']['dashboard_incoming_links'] );
unset( $wp_meta_boxes['dashboard']['normal']['core']['dashboard_right_now'] );
unset( $wp_meta_boxes['dashboard']['normal']['core']['dashboard_plugins'] );
unset( $wp_meta_boxes['dashboard']['normal']['core']['dashboard_recent_drafts'] );
unset( $wp_meta_boxes['dashboard']['normal']['core']['dashboard_recent_comments'] );
unset( $wp_meta_boxes['dashboard']['side']['core']['dashboard_primary'] );
unset( $wp_meta_boxes['dashboard']['side']['core']['dashboard_secondary'] );
remove_meta_box( 'dashboard_activity', 'dashboard', 'normal' );
}
add_action( 'wp_dashboard_setup', 'remove_dashboard_widgets' );
9 . Register Menu Location in Navigation Menu:
It is one of WordPress useful functions for register menu location. If you want to add theme location in navigation menu, you need to add following code into functions.php:
/* Include navigation menus */
function register_my_menu() {
register_nav_menu( 'nav-menu', __( 'Navigation Menu' ) );
}
add_action( 'init', 'register_my_menu' );
10 . Add Custom Logo in WordPress Admin Login Page:
It is one of WordPress useful functions to add custom logo in admin pages. If you want to display custom logo instead of default wordpress logo in wordpress admin login page, you need to add following code into functions.php
/* Insert custom login logo */
function custom_login_logo() {
echo '
<style>
.login h1 a {
background-image: url(image.jpg) !important;
background-size: 234px 67px;
width:234px;
height:67px;
display:block;
}
</style>
';
}
add_action( 'login_head', 'custom_login_logo' );
11 . Modify Admin Footer Text
It is one of WordPress useful functions for change admin footer text. If you want to change footer text on admin pages, you need to add following code into functions.php:
/* Modify admin footer text */
function modify_footer() {
echo 'Created by <a href="mailto:you@example.com">you</a>.';
}
add_filter( 'admin_footer_text', 'modify_footer' );
12 . Add New Widget Areas in WordPress
If you want to add custom widget area, add following code in functions.php file:
function scanwp_widget_area() {
$args = array(
'id' => 'scanwp_sidebar',
'name' => __( 'Special Footer Text', 'text_domain' ),
'description' => __( 'Prints out special text added from the widget page', 'text_domain' ),
'before_title' => '<div class="scanwp_sidebar">',
'after_title' => '</div>',
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
);
register_sidebar( $args );
}
add_action( 'widgets_init', 'scanwp_widget_area' );
13 . Upload More Types of File Types in WordPress
If you want to upload custom file type like svg which is restricted by default, you need to add following code into functions.php file to allow it:
function additional_myme_types($mime_types){
$mime_types['svg'] = 'image/svg+xml';
$mime_types['svgz'] = 'image/svg+xml';
//Add additional mime types here
return $mime_types;
add_filter('upload_mimes', 'additional_myme_types', 1, 1);
14: Include Scripts and Styles on Front-end:
Add Following code into functions.php file for include css and javascript:
/* Enqueue styles and scripts */
function custom_scripts() {
wp_enqueue_style( 'bootstrap', get_template_directory_uri() . '/css/bootstrap.min.css', array(), '3.3.6' );
wp_enqueue_style( 'style', get_template_directory_uri() . '/css/style.css' );
wp_enqueue_script( 'bootstrap', get_template_directory_uri() . '/js/bootstrap.min.js', array('jquery'), '3.3.6', true );
wp_enqueue_script( 'script', get_template_directory_uri() . '/js/script.js' );
}
add_action( 'wp_enqueue_scripts', 'custom_scripts' );
15 . Create Custom Thumbnail Size:
Add following code into functions.php if you want to use custom thumbnail size:
/* Create custom thumbnail size */
add_image_size( 'custom-thumbnail', 250, 250, true );
/* For Retrieve Thumbnail, Add Following code on file in which you want to display custom thumbnail */
$thumb = wp_get_attachment_image_src( get_post_thumbnail_id($post->ID), 'custom-thumbnail' );
echo $thumb[0];
16 . Custom avatar support
If you don’t particularly like the WordPress default avatar options, you can define your own by adding following code into functions.php.
if ( !function_exists('cake_addgravatar') ) {
function cake_addgravatar( $avatar_defaults ) {
$myavatar = get_template_directory_uri() . '/images/avatar.png';
$avatar_defaults[$myavatar] = 'avatar';
return $avatar_defaults;
}
add_filter( 'avatar_defaults', 'cake_addgravatar' );
}
17 . Remove WordPress Admin Bar
It is one of WordPress useful functions for remove admin bar. If you want to admin bar from top add following code into functions.php
/* Remove WordPress admin bar */
function remove_admin_bar() {
remove_action( 'wp_head', '_admin_bar_bump_cb' );
}
add_action( 'get_header', 'remove_admin_bar' );
18. Load More Pagination for custom post type
/* Add Load More button after posts on template where all posts are showing */
<?php if ( $your_custom_query_loop->max_num_pages > 1 ) { ?>
<div class="btn_holder center">
<a class="btn" id="more_posts" href="#1">Load More</a>
</div>
<?php } ?>
/* Add below scripts at bottom on template where all posts are showing */
<script>
var page = 0;
jQuery(function($) {
$('body').on('click', '#more_posts', function() {
page = page+1;
var data = {
'action': 'load_posts_by_ajax',
'query': '<?php echo json_encode( $your_custom_query_loop->query_vars ); ?>',
'page' : page,
'max_page' : <?php echo $your_custom_query_loop->max_num_pages; ?>,
'security': '<?php echo wp_create_nonce( 'load_more_posts' ); ?>'
};
$.post(
'<?php echo admin_url( 'admin-ajax.php' ); ?>', data, function(response) {
if($.trim(response) != '') {
$('#resultdata').append(response);
} else {
$('#more_posts').hide();
}
data['page']++;
if ( data['page'] == data['max_page'] )
{
$('#more_posts').hide();
}
});
});
});
</script>
/* Add Following code in functions.php file of your active theme */
<?php
/* Load More Pagination For Your posts */
function load_posts_by_ajax_callback() {
check_ajax_referer('load_more_posts', 'security');
$args = json_decode( wp_unslash( $_POST['query'] ), true );
$args['paged'] = $_POST['page'] + 1;
$args['post_status'] = 'publish';
unset($args["taxonomy"]);
unset($args["term"]);
$post = new WP_Query($args);
while ($post->have_posts()) { $post->the_post();
// Your code
}
wp_reset_postdata();
die(); // use die instead of exit
}
add_action('wp_ajax_load_posts_by_ajax', 'load_posts_by_ajax_callback');
add_action('wp_ajax_nopriv_load_posts_by_ajax', 'load_posts_by_ajax_callback');
/* End of Load More Pagination For Your posts */
?>
19. WordPress remove menus from admin dashboard:
Add following code into functions.php file of your active theme:
<?php
function remove_menus () {
global $menu;
$restricted = array(__('Dashboard'), __('Posts'), __('Media'), __('Links'), __('Pages'), __('Appearance'), __('Tools'), __('Users'), __('Settings'), __('Comments'), __('Plugins'));
end ($menu);
while (prev($menu)){
$value = explode(' ',$menu[key($menu)][0]);
if(in_array($value[0] != NULL?$value[0]:"" , $restricted)){
unset($menu[key($menu)]);
}
}
}
add_action('admin_menu', 'remove_menus');
?>
20) Customize recent posts widget:
It is one of WordPress useful functions for customize recent post widget. Add following code into functions.php file of your active theme to overwrite default recent posts widget:
<?php
/* Customize Recent Post Widget*/
Class My_Recent_Posts_Widget extends WP_Widget_Recent_Posts {
function widget($args, $instance) {
extract( $args );
$title = apply_filters('widget_title', empty($instance['title']) ? __('Recent Posts') : $instance['title'], $instance, $this->id_base);
if( empty( $instance['number'] ) || ! $number = absint( $instance['number'] ) )
$number = 10;
$r = new WP_Query( apply_filters( 'widget_posts_args', array( 'posts_per_page' => $number, 'no_found_rows' => true, 'post_status' => 'publish', 'ignore_sticky_posts' => true ) ) );
if( $r->have_posts() ) :
echo $before_widget;
if( $title ) echo $before_title . $title . $after_title; ?>
<ul>
<?php while( $r->have_posts() ) : $r->the_post(); ?>
<li>
<?php $recent_img = get_the_post_thumbnail( $post->ID, 'thumbnail', array('class' => 'mr-3') ); ?>
<a href="<?php the_permalink(); ?>" class="post_img"><?php if($recent_img) { echo $recent_img; }else{ ?><img src="<?php echo get_stylesheet_directory_uri(); ?>/images/no_thumb.jpg" alt=""><?php } ?></a>
<div class="post_body">
<span class="post_date"><i class="fa fa-calendar"></i><?php the_time('M jS, Y'); ?> </span>
<h4><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h4>
<a href="<?php the_permalink(); ?>" class="read_more">Read More</a>
</div>
</li>
<?php endwhile; ?>
</ul>
<?php
echo $after_widget;
wp_reset_postdata();
endif;
}
}
function my_recent_widget_registration() {
unregister_widget('WP_Widget_Recent_Posts');
register_widget('My_Recent_Posts_Widget');
}
add_action('widgets_init', 'my_recent_widget_registration');
/* End of Customize Recent Post Widget*/
?>
21. Display Popular Posts Without Plugin:
It is one of WordPress useful functions for display popular posts without using plugin.
/* Add Following code in functions.php of your active theme */
<?php
function custom_count_post_visits() {
if( is_single() ) {
global $post;
$views = get_post_meta( $post->ID, 'my_post_viewed', true );
if( $views == '' ) {
update_post_meta( $post->ID, 'my_post_viewed', '1' );
} else {
$views_no = intval( $views );
update_post_meta( $post->ID, 'my_post_viewed', ++$views_no );
}
}
}
add_action( 'wp_head', 'custom_count_post_visits' );
?>
/* You need to add following code in page where you want to display popular posts */
<?php
$popular_posts_args = array(
'posts_per_page' => 3,
'meta_key' => 'my_post_viewed',
'orderby' => 'meta_value_num',
'order'=> 'DESC'
);
$popular_posts_loop = new WP_Query( $popular_posts_args );
while( $popular_posts_loop->have_posts() ):
$popular_posts_loop->the_post();
// Loop continues
endwhile;
wp_reset_query();
?>
WordPress useful functions provide a wealth of tools to streamline and enhance your development process. By mastering template tags, WP_Query, actions, filters, security functions, utility functions, and caching functions, you can unlock the full potential of the WordPress CMS.
These WordPress useful functions empower you to customize themes, simplify queries, extend functionality, secure your site, optimize performance, and deliver exceptional user experiences. Embrace the power of WordPress useful functions and elevate your development workflow to new heights.
I Hope this helps you to know WordPress Useful Functions!