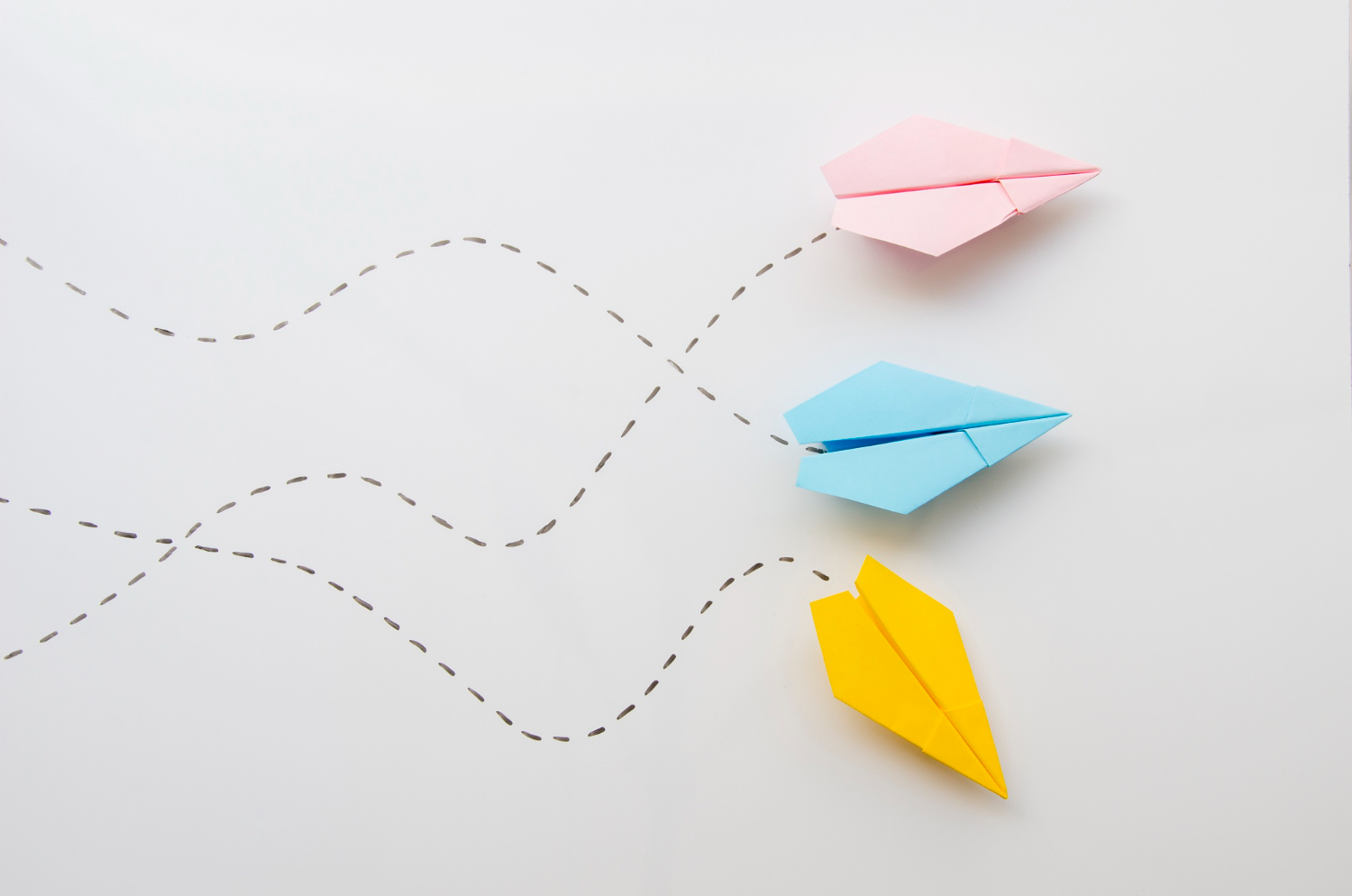
In this article, we’ll learn about React Router.
Table of Contents
Efficient navigation is essential in the massive web development world to create user-friendly experiences. The React Router is one of the strongest tools that has become a basic element for navigation in responsive applications.
We will begin a journey through the complicated features of React Router, as well as its structure and best practices on how to create consistent and dynamic navigation systems within your applications in this blog post.
What is a React Router:
React Router is a popular library for managing client-side routing in a React application. It allows developers to define and organize different routes in the app, and map them to specific components that will be rendered when a user navigates to a certain URL. This gives the app a more seamless and intuitive user experience, similar to how a single-page application (SPA) behaves.
React Router uses a component called a <Router>
to keep track of the current URL and to render the appropriate components based on that URL. The <Router>
component is usually wrapped around the entire application, and it can have one or more <Route>
components as children. Each <Route>
component maps a specific URL path to a component that should be rendered when that path is visited.
When a user navigates to a URL, React Router will match the URL’s path against the path specified in each <Route>
component. When it finds a match, it will render the component associated with that route. If no match is found, it will render a 404
component or the component specified in the not-found
prop of the <Router>
component.
React Router also provides other components such as <Link>
, <NavLink>
, <Switch>
that can be used to create links, highlight the active link, and handle cases where multiple routes match the current URL respectively.
React Router provides a powerful and flexible API for defining routes, handling dynamic parameters, managing browser history, and more. It also includes built-in support for handling common use cases such as redirecting and protecting routes.
React Router is often used in conjunction with other libraries such as Redux to manage the application’s global state. It is also considered as one of the most popular routing libraries for React, and it is widely used and well-supported by the React community.
Installation:
Before installation, make sure you have pre-installed node js or yarn.
First, you need to install the react-router-dom package, a set of bindings for react-router designed for web applications. You can do this using npm or yarn:
npm install react-router-dom
# or
yarn add react-router-dom
To use React Router in your application, you’ll need to import the BrowserRouter
, Route
, and Link
components. These components are used to define the routes and links that will be used in your application.
How to use React Router with Example:
Create the components for the different pages or views of your application. Here, we create two files one for the homepage and another for the About page. Each page or view should be a separate React component. See the below example where we create two component files called “home.js” and “about.js” files inside the components directory.
// components/Home.js import React from 'react'; const Home = () => { return <div>Home Page</div>; }; export default Home; // components/About.js import React from 'react'; const About = () => { return <div>About Page</div>; }; export default About;
In your main application file (usually App.js), set up the router. You typically use the Routes to provide routing functionality to your application.
// App.js import React from "react"; import { Routes, Route } from "react-router-dom"; import Home from './components/Home'; import About from './components/About'; function App() { return ( <Routes> <Route path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes> ); } export default App;
In this example, we’ve defined two routes: one for the home page ("/"
) and one for the About page ("/about"
).
To navigate between these pages, you can use Link
components from react-router-dom
. These links ensure that navigation happens without a full page reload.
// components/Header.js import React from 'react'; import { Link } from 'react-router-dom'; const Header = () => { return ( <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> </ul> </nav> ); }; export default Header;
Include your navigation links (e.g., in a header or sidebar) in your application to allow users to navigate between pages.
// App.js import React from "react"; import { Routes, Route } from "react-router-dom"; import Home from "../components/Home"; import About from "../components/About"; import Header from "../components/Header"; const App = () => { return( <> <Header /> <Routes> <Route path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes> </> ); }; export default App;
Also, you need to import BrowserRouter in the main index.js file from where react code is injected into the HTML root element. see below for the updated code
import React, { StrictMode } from "react"; import { createRoot } from "react-dom/client"; import { BrowserRouter } from "react-router-dom"; import App from "./App"; const rootElement = document.getElementById("root"); const root = createRoot(rootElement); root.render( <StrictMode> <BrowserRouter> <App /> </BrowserRouter> </StrictMode> );
Now, you have a basic navigation router set up in your React application using react-router-dom
. Users can navigate between the home and about pages using the provided links, and the content will change dynamically without a full page reload. You can expand upon this foundation to create more complex routing structures and integrate additional features as needed for your application.
You can whether ck example code here: https://github.com/umangit/react-routing-example.git
Credits:
- Image by Freepik
References:
- https://reactrouter.com/en/main/start/tutorial
- https://www.quora.com/unanswered/What-is-React-Router-1
Conclusion:
Lastly, the React Router is a powerful tool that allows React application users to navigate easily. You can create a more dynamic and user-friendly experience for your website applications by mastering its features.
React Router will be a valuable ally as you continue to learn the ever-changing web development landscape, whether you are designing your Portfolio site or developing an advanced Web application. Happy routing!
Pretty! This was a really wonderful article. Thank
you for supplying this info.